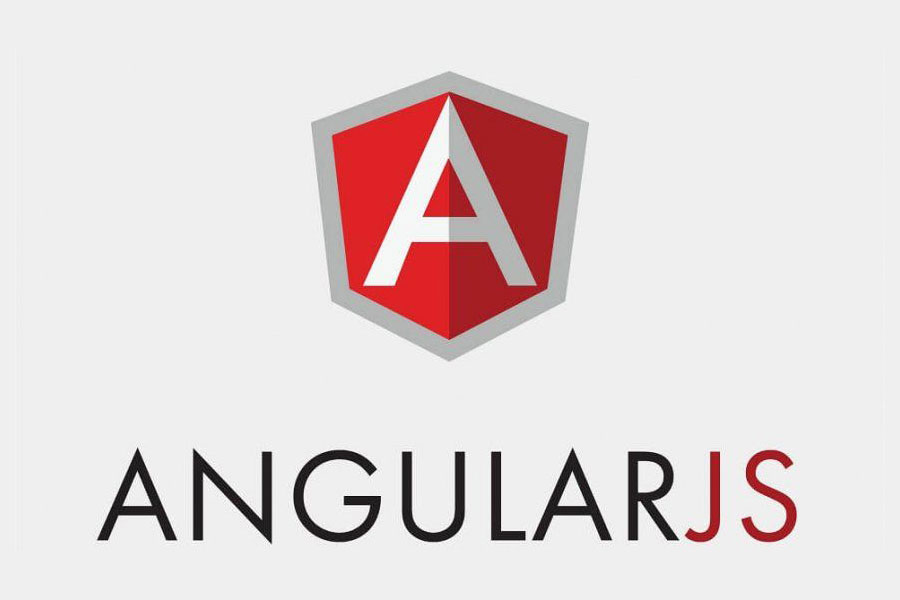
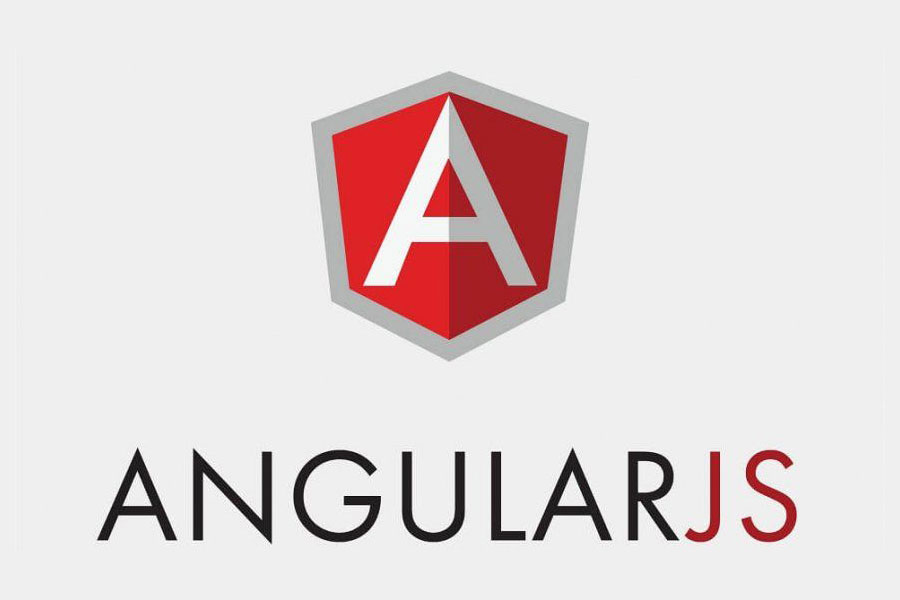
Dependency Injection mechanism in angularJS
- 25-07-2022
- Toanngo92
- 0 Comments
DI is a powerful angularJS mechanism, it helps to inject an object defined from a 3rd party library into the angular application, which is passed as an element in the array as the 2nd parameter when instantiating the angularJS module, based on the way the code we understand, it helps to refer to the library object but without using direct reference statement, is the technique of making a function depend on the module at execution time without coding it .
See more about dependency injection at wikipedia: https://en.wikipedia.org/wiki/Dependency_injection
Programmers can divide the application into many different components (component), and can inject from one component to another, the modularization mechanism in angularJS application makes it easy to configure, reuse, update Update and test components. The builtin injector (default injected object) will be responsible for creating components (components), sorting out dependencies, providing them to other components when required.
As mentioned, injection mechanism makes the code more reusable, for example if we want to create a feature that needs to be reused in several different situations in the application, think of defining a business. common services and reuse them by injecting them as a dependency.
Some of the components and objects that angularJS injects into the application through the injection mechanism:
- value
- Provider
- Constant
- Factory
- Service
Mục lục
Value
This is a method of the module, it helps the programmer to define an object (object), string(number), or number(number) and inject them into the controller during the config phase (bootstrapping phase). . It is often used to inject values into server, controller, facetory.
See the example below:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Dependency injection</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> </div> </body> <script> var myApp = angular.module('myApp', []); myApp.value("number",10); myApp.value("string","Hello"); myApp.value("object",{name:"Nguyen Van A",age:20}); myApp.value("array",["Nguyen Van A","Nguyen Van B","Nguyen Van C"]); myApp.controller('myController', function($scope,number,string,object,array){ $scope.number = number; $scope.string = string; $scope.object = object; $scope.array = array; console.log($scope.number); console.log($scope.string); console.log($scope.object); console.log($scope.array); }); </script> </html>
Result when running the program:
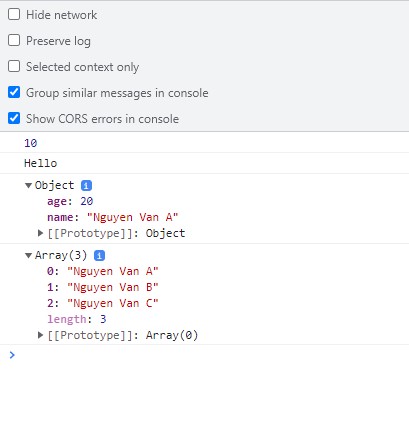
Provider
AngularJs uses the provider() method to create a service or factory during the configuration phase (when starting to launch and configure the application), a provider is a separate factory function along with the $get() and return value, factory or service (it’s a bit confusing, but look down the example. It tells angualrJS how to create an injectable service (the business is injectable, so the provider defines the service and is created through the service) builtin is $provide
Consider the example below:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example Provide</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.32/angular.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myCtrl"> <button ng-click="DisplayProvider()" ng-disabled="flag">Display</button> <div ng-bind="ProviderOutput"></div> </div> </body> <script> var app = angular.module('myApp', []); app.provider("myProvider", function () { this.$get = function () { return { Display: function () { return "Display from services"; } }; }; }); app.controller('myCtrl', function ($scope, myProvider) { $scope.ProviderOutput = "Provider outoput"; $scope.DisplayProvider = function () { $scope.flag = true; $scope.ProviderOutput = myProvider.Display(); } }); </script> </html>
This is the most basic example of how to use the provider to create injectable services into the controller, as we can see, the Display method of myProvider can already be called, and the myProvider object is injected into the controller for use, similar to other injectable objects.
In addition, we can still define functions through the app.config(), app.run() methods (methods that will run when the application config starts or the application starts running), then functions are also injectable via the injection mechanism.
Constant
In angularJS, the config() method cannot pass an instance of the service or the value defined through the value() method into it as a parameter, so it is not possible to inject a value or an entity into it. . However, it is still possible to inject a constant as a service provider during config. This means that the constant helps pass values during config, and it can also be used while the application is running (run time). )
Prove it through an example:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Dependency injection</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> </div> </body> <script> var myApp = angular.module('myApp', []); myApp.value("testnumber",100); myApp.config(function($provide,testnumber){ console.log(testnumber); }); myApp.controller('myController', function($scope,testnumber){ }); </script> </html>
After running, the browser gives an error:
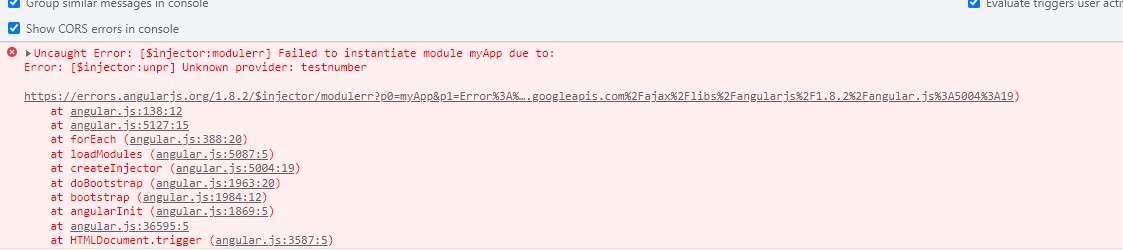
for constant, we can inject value into config() or run(), even change the value. However, it doesn’t make too much sense because even though the value of the constant has been changed in the config, the controller layer and the variable’s data are still identified by value when initializing (constant is a constant, no change in value. value throughout the program) See the example below:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Dependency injection</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> {{testnumber}} </div> </body> <script> var myApp = angular.module('myApp', []); myApp.constant("testnumber",100); myApp.config(function($provide,testnumber){ console.log($provide); testnumber = 1000; console.log(testnumber); }); myApp.controller('myController', function($scope,testnumber){ $scope.testnumber = testnumber; }); </script> </html>