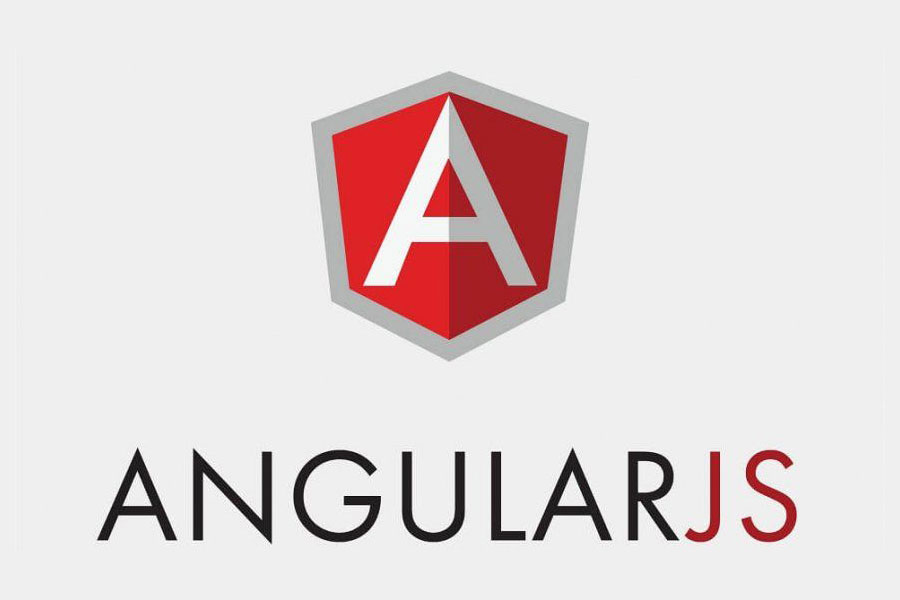
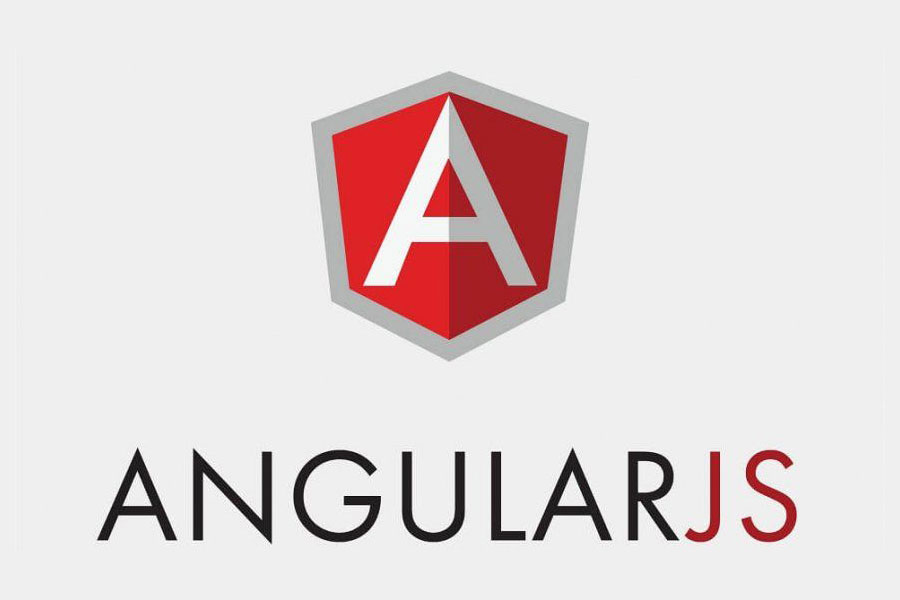
Directive in angularJS and the list of directives
- 24-07-2022
- Toanngo92
- 0 Comments
As we have approached, angularJS is known as a framework used to build web applications, that is, provided with a number of pre-built structures that help us to develop applications easily and coherently. more.Directives is one of the most important components of angularJS, helping us to inherit basic HTML elements, making html interface templates reusable, easy to test.
Mục lục
AngularJS Directives Concepts
Directives (directives) is a behavior that will be triggered when a specific construct is encountered during AngularJS compilation. Read more in-depth about angularJS HTML Compiler here: https://docs.angularjs.org/guide/compiler
The following example demonstrates 2 ways to use ng-bind directive via attribute or class:
<!DOCTYPE html> <html lang="en" ng-app="exampleviewApp"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example View AngularJS</title> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script> </head> <body ng-controller="mainController"> <h2>Hello {{name}}</h2> <input type="text" ng-model="name" ng-change="change()" /> <span class="ng-bind: name;"></span> </body> <script> var exampleviewApp = angular.module('exampleviewApp', []); exampleviewApp.controller('mainController', function($scope) { $scope.name = ''; $scope.length = 0; }); </script> </html>
Directives builtin are usually structured as an attribute of HTML, (which can be written in class or tag style), angular recognizes these special attributes and we can imagine it as giving display when using these attributes, so it is necessary to comply with Angular's own characteristics and its principle that the starting letter is always the ng character, following the ng-directivename example structure, where directivename is the name of the directive we use. As in the previous examples, to initialize the application, to declare it as a Directive Controller, we declare ng-controller, declare a directive model we declare ng-model, or let data binding we use ng-bind…
A few notes:
- ng-app must always be defined, and for versions after legacy, must always name ng-app and declare it explicitly.
- In the html structure, there is always only 1 ng-app, there can be many other ng directives.
- Directives list documentation: https://docs.angularjs.org/api/ng/directive
Matching Directives (matching directives)
List of popular AngularJS Directives
ng-app
Docs: https://docs.angularjs.org/api/ng/directive/ngApp
When we declare a Directive ng-app, AngularJS will understand that starting an Angular application, it will identify this as the root element and next will initialize the internal configuration parameters that we call are bootstraps. In addition, ng-app is also used to describe various modules in the application. As for the example, we have already demoed a lot, so I won't talk about it any more.
ng-init
Docs: https://docs.angularjs.org/api/ng/directive/ngInit
ng-model
Docs: https://docs.angularjs.org/api/ng/directive/ngModel
Use ng-model directive used to bind (bind) data inside form input tags like input, select, textarea to the properties of the $scope variable on the controller layer.
ng-model directives provide some of the following features:
- Provides validation of data types for the application (number, email, required)
- Provides status for application data (invalid,dirty,touch,error)
- Provide css class for HTML element (will be discussed in post validate form)
- Bind an element's data to HTML Forms
ng-repeat
Docs: https://docs.angularjs.org/api/ng/directive/ngRepeat
Use ng-repeat directive to be able to loop through HTML elements when you have a list model, be it array or object, these are very useful and frequently used directives that support iterate tags directly in HTML, which pure javascript cannot do.
Example of ng-repeat
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example ng-repeat</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="mainController"> <table> <thead> <tr> <th>Name</th> <th>Country</th> </tr> </thead> <tbody> <tr ng-repeat="item in names"> <td>{{item.name}}</td> <td>{{item.country}}</td> </tr> </tbody> </table> <ul> <li ng-repeat="item in listnotes">{{item.id}} - {{item.title}} - {{item.content}}</li> </ul> </div> </body> <script> var myApp = angular.module('myApp', []); myApp.controller('mainController', function ($scope) { $scope.names = [ { name: 'Jani', country: 'Norway' }, { name: 'Hege', country: 'Sweden' }, { name: 'Kai', country: 'Denmark' } ]; $scope.listnotes = [ {'id': 1, 'title': 'Note 1', 'content': 'Content 1'}, {'id': 2, 'title': 'Note 2', 'content': 'Content 2'}, {'id': 3, 'title': 'Note 3', 'content': 'Content 3'} ] }); </script> </html>
ng-hide / ng-show
Use ng-hide when we want to hide HTML element in a specific condition, in contrast to ng-hide ng-show, useful in multi-step crawling SPA application situation, for example The example below is a use case of ng-hide for creating a multi-step user data collection survey:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>ng-hide/ng-show example</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-app="surveyApp"> <div ng-controller="mainController"> <h1>Survey Form</h1> <form name="surveyForm" ng-submit="handleSubmit()"> <div ng-hide="step1"> <div> <label>What is your name ?</label> <input type="text" ng-model="user.name" required /> </div> <div> <label>How old are you ?</label> <input type="number" placeholder="" ng-model="user.age" required /> </div> <div> <label>Do you get married ?</label> <input type="radio" ng-model="user.married" value="yes" />Yes <input type="radio" ng-model="user.married" value="no" />No </div> <div> <label>What kind of job are you doing ?</label> <select ng-model="user.job"> <option value="developer">Developer</option> <option value="teacher">Teacher</option> <option value="businessconsult">Business Consultant</option> <option value="other">Other</option> </select> </div> <button type="button" data-step="1" ng-click="nextstep($event)">Next step</button> </div> <div ng-hide="step2"> <div> <label>What is your favorite sports ?</label> <input type="text" ng-model="interest.sports" required /> </div> <div> <label>What is your favorite music ?</label> <input type="text" ng-model="interest.music" required /> </div> <div> <label>What is your favorite movies ?</label> <input type="text" ng-model="interest.movies" required /> </div> <div> <label>What is your favorite books ?</label> <input type="text" ng-model="interest.books" required /> </div> <div> <label>What is your favorite games ?</label> <input type="text" ng-model="interest.games" required /> </div> <button type="button" data-step="2" ng-click="prevstep($event)">Previous step</button> <button type="button" data-step="2" ng-click="nextstep($event)">Next step</button> </div> <div ng-hide="step3"> <div> <label>Please provide your salary ?</label> <input type="text" ng-model="finances.salary" required /> </div> <div> <label>Please provide your savings ?</label> <input type="text" ng-model="finances.savings" required /> </div> <div> <label>Please provide your investments ?</label> <input type="text" ng-model="finances.investments" required /> </div> <div> <label>Please provide your debt ?</label> <input type="text" ng-model="finances.debt" required /> </div> <button type="button" data-step="3" ng-click="prevstep($event)">Previous step</button> <button>submit form</button> </div> <div ng-hide="step4"> Thanks for your submission Let us show what you provide: <br /> Your name: {{user.name}} <br /> Your age: {{user.age}} <br /> Your job: {{user.job}} <br /> Your favorite sports: {{interest.sports}} <br /> Your favorite music: {{interest.music}} <br /> Your favorite movies: {{interest.movies}} <br /> Your favorite books: {{interest.books}} <br /> Your favorite games: {{interest.games}} <br /> Your salary: {{finances.salary}} <br /> Your savings: {{finances.savings}} <br /> Your investments: {{finances.investments}} <br /> Your debt: {{finances.debt}} <br /> Thanks for contact us ! </div> </form> </body> <script> var surveyApp = angular.module('surveyApp', []); surveyApp.controller('mainController', ($scope) => { $scope.step1 = false; $scope.step2 = true; $scope.step3 = true; $scope.step4 = true; $scope.user = { name: 'sdfsdf', age: '', married: 'no', job: 'developer', } $scope.interest = { sports: '', music: '', movies: '', books: '', games: '', } $scope.finances = { salary: 0, savings: 0, investments: 0, debt: 0, } $scope.prevstep = ($event) => { switch ($event.target.dataset.step) { default: case '2': $scope.step2 = true; $scope.step1 = false; break; case '3': $scope.step3 = true; $scope.step2 = false; break; } if ($event.target.dataset.step == 1) { } else if ($event.target.dataset.step == 2) { } } $scope.nextstep = ($event) => { switch ($event.target.dataset.step) { default: case '1': $scope.step1 = true; $scope.step2 = false; break; case '2': $scope.step2 = true; $scope.step3 = false; break; case '3': $scope.step3 = true; $scope.step4 = false; break; } if ($event.target.dataset.step == 1) { } else if ($event.target.dataset.step == 2) { } } $scope.handleSubmit = () => { // handle submit to send data to server or send email ... $scope.step3 = true; $scope.step4 = false; console.log($scope.user); console.log($scope.interest); console.log($scope.finances); } }); </script> </html>
ng-include
By default, HTML does not provide a solution to be able to embed the content of one HTML page in another, but with angularJS, this problem is solved through the ng-include directive, we can completely embed an HTML page directly. HTML file into another file, useful when the HTML structure is too long, we can split it into smaller files to easily maintain and update the code for each component, minimizing errors arising in the development process. application.
Example of ng-include, passing data from controller to include file to traverse through onload attribute, however this is not a good way to use, because there is problem with scoping, in this situation we should use custom directive (directive self-defined) will be approached below.
See more documents:
Step 1: create file index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example ng-include</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body> <div ng-app="myApp"> <div ng-controller="myController"> <h1>ng-include example</h1> <div ng-include="'table.html'" onload="items = items"></div> </div> </div> </body> <script> var myApp = angular.module('myApp', []); myApp.controller('myController', function($scope) { $scope.items = [ {name: 'Jani', country: 'Norway'}, {name: 'Hege', country: 'Sweden'}, {name: 'Kai', country: 'Denmark'} ] }); </script> </html>
Step 2: create file table.html
<table> <tr ng-repeat="item in items"> <td>{{item.name}}</td> <td>{{item.country}}</td> </tr> </table>
Results after printing to the screen
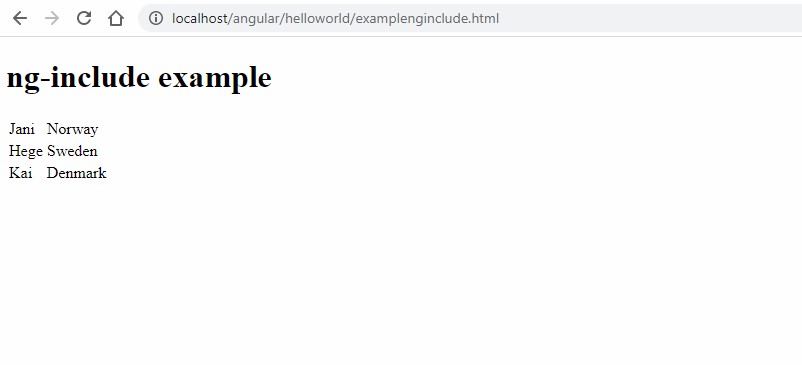
ng-form / ng-submit
Docs: https://docs.angularjs.org/api/ng/directive/ngForm
With creating a form in pure HTML to submit data to the server, we use the <form> tag and handle the submit event via the ng-submit directive, but we reach for the ng-form directive because of this directive. Directly bind to ngForm object on script layer, and this object stores attributes used in data validation, but when creating html tag, we still create normal <form> tag, you can read more docs of angularJS. To handle the submit event, we use the ng-submit directive. There is a point we notice here, if you learn pure HTML and JS, by default after submitting, the website will be reloaded, but not with this ng-submit, because as I said, angularJS uses to build a SPA application, so after submitting, the data will be put in a list, and we use ajax request to send the data to the server without reloading the page.
Example of ng-form combined with ng-submit :
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example ngform</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="mycontroller"> <form ng-submit="submit()"> <input ng-model="myForm.name" type="text" name="name" placeholder="Name"> <input ng-model="myForm.email" type="email" name="email" placeholder="Email"> <input ng-model="myForm.password" type="text" name="password" placeholder="password"> <input ng-model="myForm.confirmPassword" type="text" name="confirmpassword" placeholder="confirmpassword"> <input type="submit" value="Register"> </form> </div> </body> <script> var app = angular.module('myApp', []); app.controller('mycontroller', function($scope) { $scope.myForm = {}; $scope.myForm.name = "sdfsdf"; $scope.myForm.email = ""; $scope.myForm.password = ""; $scope.myForm.confirmPassword = ""; $scope.submit = function() { console.log($scope.myForm); // send ajax to server or database // in this case, we just save data to localstorage localStorage.setItem('listuser', JSON.stringify($scope.myForm)); } }); </script> </html>
Define your own Directives in angularJS (custom directives)
Docs: https://docs.angularjs.org/guide/directive
Although angularJS provides a lot of directives that help us to meet most of the requirements when building an application, we can create a self-defined directive to meet the business in the case of directives. default is not met.
.AngularJS application on startup will automatically find matching elements and perform one time operation using custom directive's compile() method, then process the element using link method () of a custom directive based on the directive's scope. Before going into the example, we need to understand the general concept of directives type.
- Element directives − Directives that fire when a matching element is encountered.
- Attribute − The directive that fires when a matching attribute is encountered.
- Class (class directive) − A directive that fires when a matching class is encountered.
- Comment (comment directive) − The directive is activated when a matching comment is encountered.
These directives type are used to specify the value for the restrict attribute when initializing directives: E (Element) A(Attributes) C (Class) M (comment). Normally, we mainly use EA (Element and Attribute indicator).
Example of self-initiating a basic custom directive in angularJS
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example custom directive</title> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> <customdirective></customdirective> <div customdirective></div> <div class="customdirective"></div> </div> </body> <script> var app = angular.module('myApp', []); app.directive('customdirective', function() { return { restrict: 'EAC', template: '<h2>Hello World custom directive</h2>' }; }); app.controller('myController', function($scope) { $scope.name = ""; }); </script> </html>
Results when printing to the browser
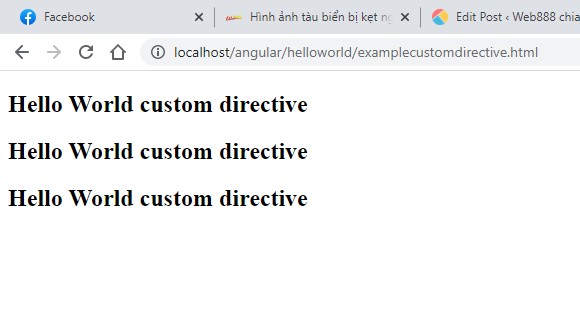
For example, creating an application with multiple directives, each directive contains parameters passed to increase the flexibility of the program, with this feature, we can create our own, reusable HTML structures and The data is dynamically updated according to the input parameter.
Step 1: create the sample index.html code file as follows
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example custom directive</title> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> <form ng-submit="handlesubmit($event)"> <div surveyform name="person1" submit="directiveSubmit"></div> </form> <form ng-submit="handlesubmit($event)"> <div surveyform name="person2"></div> </form> </div> </body> <script> var app = angular.module('myApp', []); app.controller('myController', function ($scope) { $scope.person1 = { fullname: "John", email: "abc@gmail.com", phonenumber: 1234567890 } $scope.person2 = { fullname: "Toanngo92", email: "trienkhaiweb@gmail.com", phonenumber: 2123456789 } $scope.handlesubmit = function ($event) { console.log($event); // todo here } }); app.directive('surveyform', function () { let directive = {}; directive.restrict = 'EAC'; directive.templateUrl = 'surveyForm.html'; directive.scope = { person: '=name', submit: '=' }; directive.compile = function (element, attributes) { // console.log(element); element.css("border", "1px solid #cccccc"); element.css("padding", "10px"); //linkFunction is linked with each element with scope to get the element specific data. var linkFunction = function ($scope, element, attributes) { } return linkFunction; } return directive; }); </script> </html>
Step 2: Create surveyForm.html file with below structure
<label>fullname</label> <br /> <input type="text" ng-model="person.fullname" /> <br /> <label>Email</label> <br /> <input type="text" ng-model="person.email" /> <br /> <label>Phone</label> <br /> <input type="number" ng-model="person.phonenumber" /> <br /> <button type="submit">submit</button>
Result when printing to the browser:
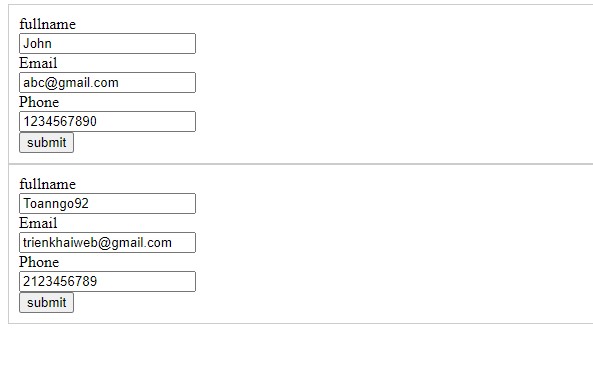
We can see that we can reuse 2 html forms without rewriting, 2 forms running the same structure and using 2 separate models.
Here is the end of the basic concepts of directives, in fact, if using the default directives is already enough in the learning process, for complex projects that need to reuse many components, we can use them. solution apply custom directives to solve.