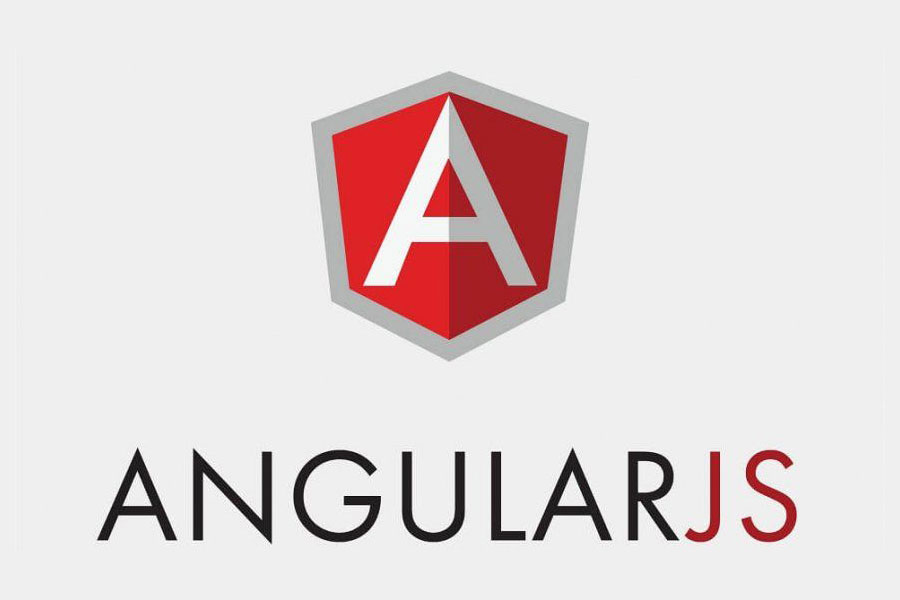
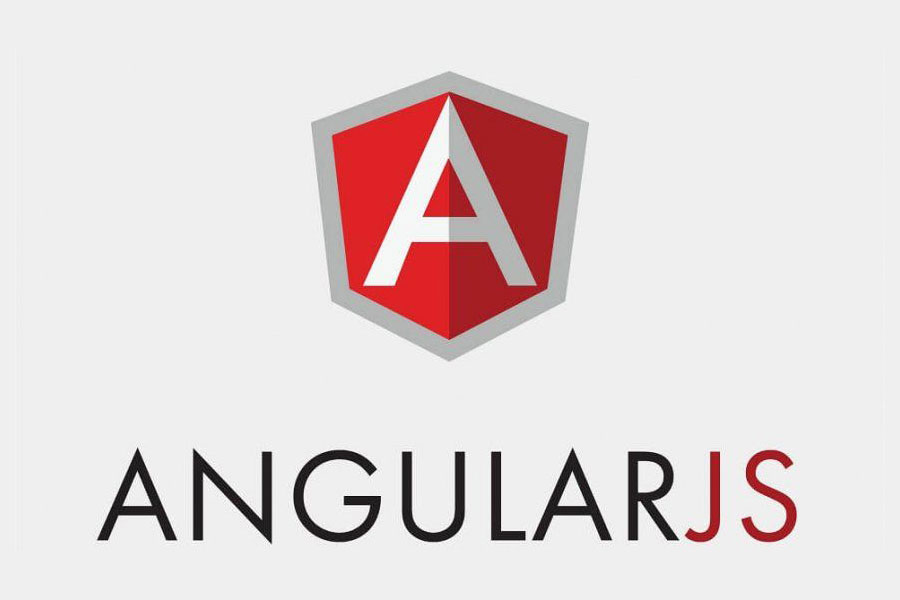
Filter (filter) in angularJS
- 25-07-2022
- Toanngo92
- 0 Comments
Mục lục
The concept and role of filters in angularJS
When approaching with pure javascript, we see, when working with data, there are some situations where we will have to convert a rather complicated persistent data type, to take a real example, when we have a variable representing the amount with data type number (float) , if we want to print to the screen in currency format, we will have to do the following steps sequentially:
- Convert data type to string,
- Concatenate strings by adding a $ sign after the string
- Print the string out inside the HTML content (via the innerText property)
- Repeat the above steps sequentially when the data is updated
With angularJs, we have a filter mechanism to support the model to shorten these tasks, the model does not need to convert the data type, filters will do the work of filtering the data and printing the results as we want. . In short, filters reformat data when presented to the end user
Syntax to use filters in angularJS:
{{expression | filter}}
Filter is injected into the expression via the “|” character. , followed by filter
Filters can be connected as consecutive links, for example:
{{expression | filter | filter}}
In this case, the first filter is used to filter the expression, the second filter continues to filter the value of the first filter.
Note: filter does not change the actual value of the variable, it only changes the end-user display format.
List of built-in filters in angularJS
Some of the popular built-in filters in angularJS:
- Currency
- Date
- Lowercase
- Uppercase
- Search
- …
Currency filter
Used to format the currency for the expression, which will be displayed to the user in the currency format
Docs: https://docs.angularjs.org/api/ng/filter/currency
By default, it will use the format USD Dollars with 2 numbers after the . The example below describes some ways to use filter, at html template layer and script layer, we can use filter under html layer or filter on javascript layer, but html layer way will be more common:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> {{price | currency:'$ '}} <br/> {{price | currency: '$ ' : 0}} <br/> {{price2 | currency:'VND '}} <br/> <input type="number" ng-model="price3" /> <br/> {{changeprice()}} </div> </body> <script> var app = angular.module('myApp',[]); app.controller('myController',($scope,$filter) => { $scope.price = 1000; $scope.price2 = 2000; $scope.price3 = 4000; $scope.changeprice = function(){ bind_model = $filter('currency')($scope.price3, '$ ', 5); return bind_model; } }); </script> </html>
Result when printing to the browser:
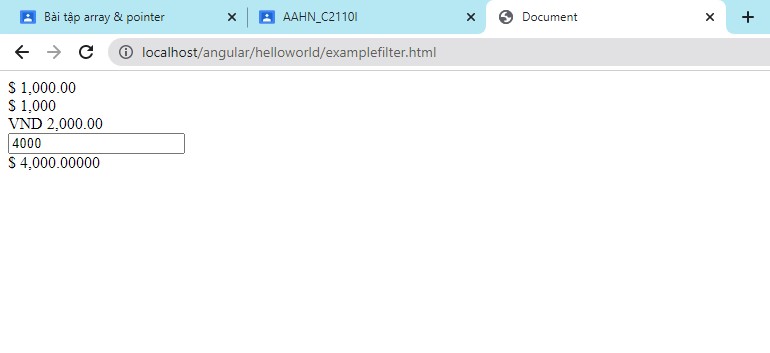
However, from my point of view, this filter does not meet VND, because the currency of my country is displayed, but VND is on the right of the number, so this filter will not respond. Of course there is a solution, we can define our own custom filter
Date filter
Docs: https://docs.angularjs.org/api/ng/filter/date
With the date filter, we can represent all date data according to the intention we want when displaying the data to the interface.
Consider the example below to better understand the date filter:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Date filter</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> {{date | date:'dd/MM/yyyy'}} </div> </body> <script> var app = angular.module('myApp',[]); app.controller('myController',($scope) => { $scope.date = Date.now(); console.log($scope.date); }); </script> </html>
Result when printing to the browser:
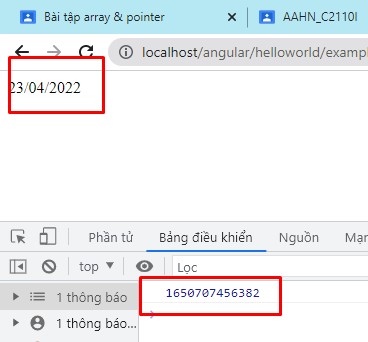
We see, the javascript layer data is timestamp representing the current time while we are writing code according to UNIX timestamp, but when printing the interface, thanks to the filter mechanism of angularJS, we have printed the date value. readable year.
Not only that, filter date has a lot of options to help you perform different formats, read on the docs to understand more.
Lowercase and uppercase
This filter is used to filter data and reformat it to lowercase or uppercase string
{{expression | lowercase}} {{expression | uppercase}}
Search Filter
Filters are used to find data in a list, which is quite convenient, see the example below to better understand the problem:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Search filter</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> <input type="text" ng-model="search" placeholder="Search"> <table> <tr ng-repeat="x in listUser | filter:search"> <td> {{x.name}} </td> <td> {{x.age}} </td> </tr> </table> </div> </body> <script> var app = angular.module('myApp', []); app.controller('myController', ($scope) => { $scope.listUser = [ { name: "toan", age: 20 }, { name: "huy", age: 21 }, { name: "hieu", age: 22 }, { name: "toan", age: 34 }, ]; }); </script> </html>
Interface when printing to the browser
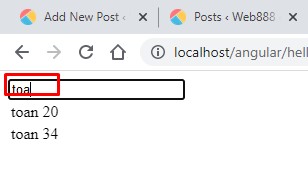
Custom Filters (self-defined filters)
If the builtin filters are not enough to use, we can completely define a custom directive to filter as we want. Syntax to initialize custom filter:
app.filter('filtername',function(){});
In the example below, I create a filter with the filter used for currency format VND
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> {{price | vndFilter}} </div> </body> <script> var app = angular.module('myApp', []); app.filter('vndFilter', function () { return function (x) { x = x.toString().replace(/B(?=(d{3})+(?!d))/g, ','); // convert float to string decimal thousand separator x += ' VND'; // concat with VND return x; }; }) app.controller('myController', function ($scope) { $scope.price = 5000000; }); </script> </html>
Results when displayed in the browser
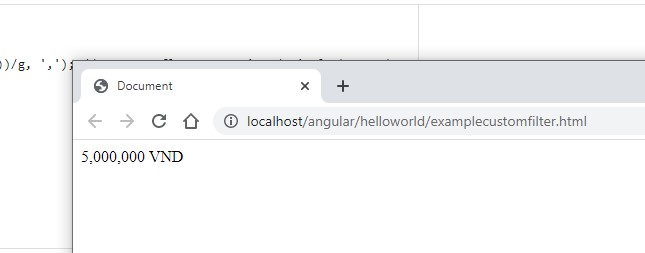