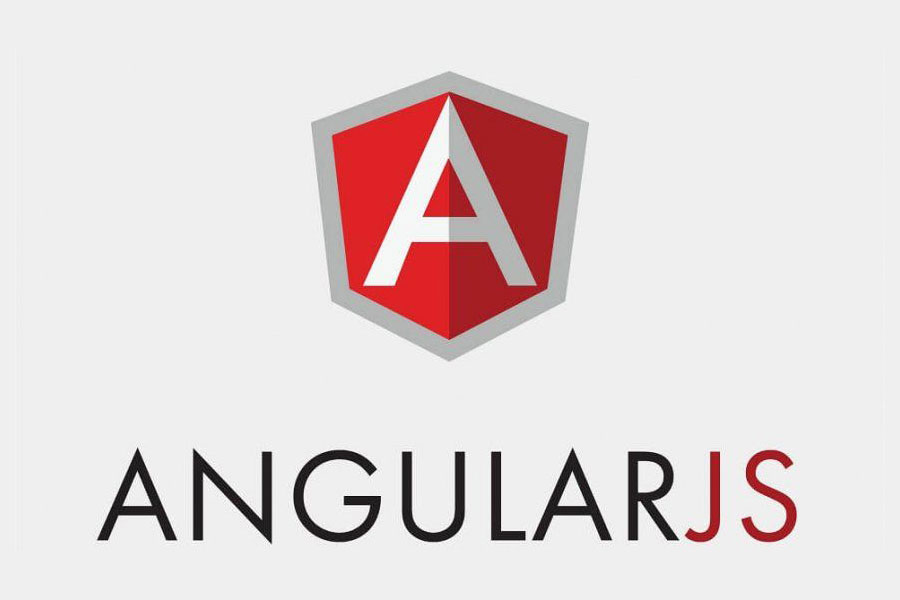
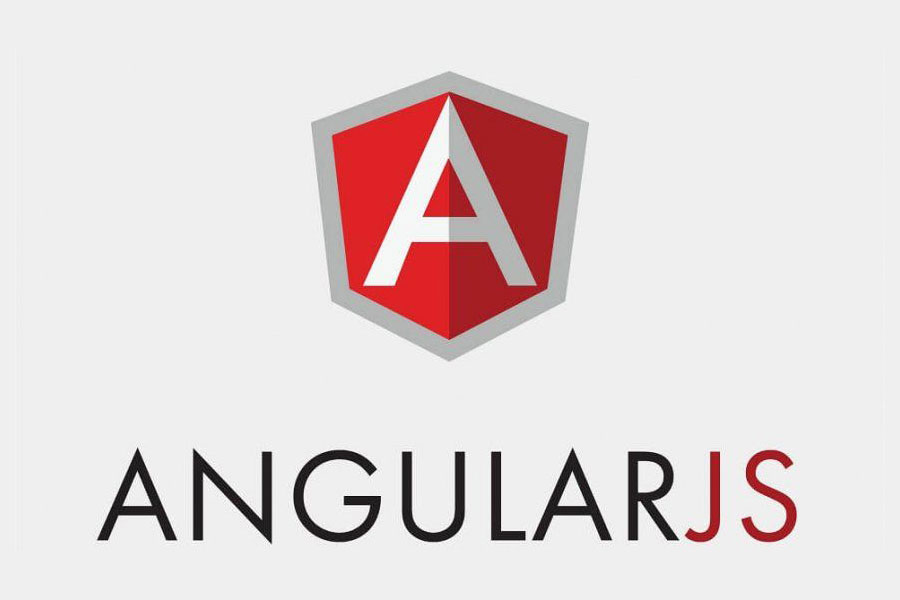
Form validation in angularJS
- 25-07-2022
- Toanngo92
- 0 Comments
Before learning this article, you must first understand the concept of the form tag in HTML, the mechanism for submitting data to the server layer, if not, go back to the HTML form article to learn.
With the form in HTML, we know that before submitting the data, the form needs to be validated to make sure the data the user enters is in the correct format before submitting it to the server. AngularJS also provides us with a mechanism to validate the form.
Mục lục
Service validate in angularJS
Docs:https://docs.angularjs.org/guide/forms
Considering the first example we have a registration form with the following fields:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Form Validate</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> <style> input{ margin-bottom: 15px; } </style> </head> <body ng-app="myApp"> <div ng-controller="myCtrl"> <form name="myForm" ng-submit="submit()"> <input type="text" ng-model="name" name="name" placeholder="Enter your name" required /> <span>{{myForm.name.$valid}}</span> <br/> <input type="email" ng-model="email" name="email" placeholder="Enter your email" required /> <span>{{myForm.email.$valid}}</span> <br/> <input type="password" ng-model="password" name="password" placeholder="Enter your password" required /> <span>{{myForm.password.$valid}}</span> <br/> <input type="password" ng-model="confirmpassword" name="confirmpassword" placeholder="Confirm your password" required /> <span>{{myForm.confirmpassword.$valid}}</span> <br/> <button>Register</button> </form> </div> </body> <script> var app = angular.module('myApp', []); app.controller('myCtrl', function($scope) { $scope.name = ""; $scope.email = ""; $scope.password = ""; $scope.confirmpassword = ""; $scope.submit = function() { console.log($scope.myForm); } }); </script> </html>
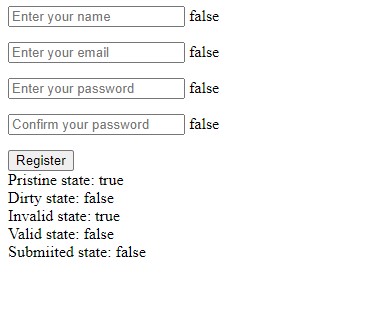
After running on the browser and entering the data, we see, by default the {{myForm.$valid}} ,… value is equal to false (meaning the form has not been validated), or {{ myForm.name.$valid}} is also updated immediately after entering a valid value, the model immediately changes to true. So we understand this $valid Service is used to check the form’s validation status, and the validation status of each input is as follows:
Form states (form states)
The following service variables represent the state of the input in the form:
- $pristine – primitive – unmodified form
- $dirty – form has been edited
- $invalid – form content is not valid
- $valid – form content is valid
- $submitted – form has been submitted
Status stream:
- pristine & invalid ( primitive field, not yet valid)
- dirty & invalid (field has been edited but is not valid)
- dirty & valid (field edited and valid)
Input states
- $untouched – untouched input field
- $touch – touch input field
- $pristine – primitive – unmodified input field
- $dirty – unedited input field
- $invalid – Invalid input field
- $valid – valid input field
These states are used to determine the user’s input state, it is completely possible to rely on the true/false value of these service states to return invalid or valid messages to the user.
CSS classes
AngularJS provides a list of builtin CSS Classes that allow programmers to style input fields based on the state of the form or input:
- ng-valid
- ng-invalid
- ng-pristine
- ng-dirty
- ng-touched
- ng-untouched
- ng-submitted
We can rely on them to css for forms to improve user experience.
Example form validation in a registration form using angularJS:
Step 1: initialize the index.html file with the following structure:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example validate form and submit</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Examole Routes AngularJS</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> <script src="https://code.angularjs.org/1.8.2/angular-route.min.js"></script> <style> .container { max-width: 400px; margin: 0 auto; padding: 15px; align-items: center; } .flex-wrap { display: flex; flex-wrap: wrap; } .list-none { list-style: none; } .menu-list { padding: 0px; margin: 0px; justify-content: flex-end; } .menu-list li { margin: 0px 5px; } .menu-list li a { text-decoration: none; color: #000; } .menu-list li a:hover { color: #46b8e6; } .no-margin { margin: 0; } .main-header { display: flex; background: #f5f5f5; } .logo-wrap { width: 45px; } .main-header nav { width: calc(100% - 46px); } .main-footer { background: #fa726c; } .white { color: #fff; } .blue { color: #46b8e6; } .form-wrap{ margin-top: 15px; } .form-wrap > *{ display: block; width: 100%; margin-bottom: 5px; } .form-wrap > input{ border: 1px solid #e1e1e1; height: 36px; line-height: 36px; padding: 5px; box-sizing: border-box; } .form-wrap > input:focus{ outline: none; } .w100{ width: 100%; } .button{ background: #ffc856; color: #fff; transition: all 0.3s; border: none; height: 40px; line-height: 40px; cursor: pointer; } .button:hover{ background: #47b7e5; color: #fff; } label{ font-weight: bold; } .error{ color: red; } input.ng-invalid{ border: 1px solid red; } input.ng-valid{ border: 1px solid green; } </style> </head> <body ng-app="myApp"> <div ng-controller="headerController" ng-class="[gc.container, gc.mainheader ,gc.flexwrap]"> <div ng-class="gc.logowrap"> <h2 ng-class="[gc.nomargin, gc.blue]">{{logotext}}</h2> </div> <nav> <ul ng-class="[gc.flexwrap , gc.listnone, gc.menulist]"> <li><a ng-href="#!">Home</a></li> <li><a ng-href="#!about">About</a></li> <li><a ng-href="#!contact">Contact</a></li> </ul> </nav> </div> <div ng-controller="mainController" ng-class="[gc.container, gc.mainbox, gc.flexwrap]"> <div ng-class="gc.w100" ng-view></div> </div> <div ng-controller="footerController" ng-class="[gc.container,gc.flexwrap,gc.mainfooter,gc.white]"> {{copyRight}} </div> </body> <script> var app = angular.module('myApp', ['ngRoute']); app.config(function ($routeProvider) { $routeProvider. when('/', { templateUrl: 'templates/register.html' }) .when('/about', { template: '<div><h2>{{aboutdata.title}}</h2><p>{{aboutdata.description}}</p></div>' }) .when('/contact', { template: "<div><h2>{{contactdata.title}}</h2><p>{{contactdata.description}}</p></div>" }); }); app.run(function ($rootScope) { // global class variable $rootScope.gc = { container: 'container', mainheader: 'main-header', mainfooter: 'main-footer', flexwrap: 'flex-wrap', listnone: 'list-none', menulist: 'menu-list', nomargin: 'no-margin', white: 'white', logowrap: 'logo-wrap', blue: 'blue', formwrap: 'form-wrap', w100: 'w100', button: 'button', error: 'error' }; }); app.filter('validmessage', function () { return function (input) { if (input == true) { return 'All data validated'; } else { return 'Please enter valid data'; } } }); app.controller('headerController', function ($scope, $location) { $scope.navigation = [ { name: 'Home', url: '/', controller: 'mainController' }, { name: 'About', url: '/about', controller: 'mainController' }, { name: 'Contact', url: '/contact', controller: 'mainController' } ]; $scope.logotext = 'web888.vn'; }); app.controller('mainController', function ($scope, $location,$rootScope) { $scope.homedata = { title: 'Home', description: 'This is home page' }; $scope.aboutdata = { title: 'About', description: 'This is about page' }; $scope.contactdata = { title: 'Contact', description: 'This is contact page' }; $scope.submit = function() { console.log(this.registerForm); alert('submited'); }; }); app.controller('footerController', function ($scope) { $scope.copyRight = '@copyright web888.vn'; }); </script> </html> </body> </html>
Step 2: initialize the file register.html inside the template folder (the templates folder is the same level as the index.html file structure):
<form name="registerForm" ng-class="gc.w100" ng-submit="submit()"> <h2 ng-class="gc.nomargin">Register Account</h2> <div ng-class="gc.formwrap"> <label>Username</label> <input type="text" ng-model="username" placeholder="username" ng-class="gc.input" required minlength="5"/> <label>Password</label> <input type="password" ng-model="password" placeholder="password" ng-class="gc.input" required minlength="5" /> <label>Confirm Password</label> <input type="password" ng-model="confirmpassword" placeholder="confirmpassword" ng-class="gc.input" required minlength="5" /> <button ng-class="gc.button">Register</button> <label>{{registerForm.$valid | validmessage}}</label> </div> </form>
Interface after running the application:
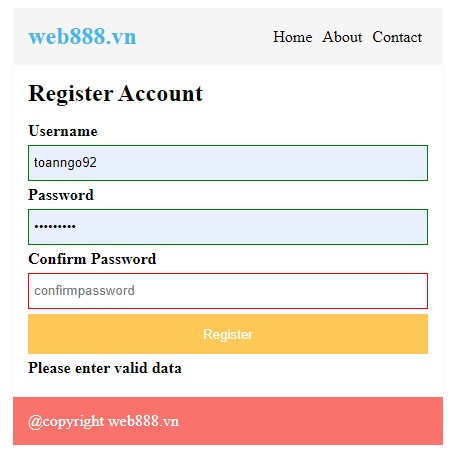