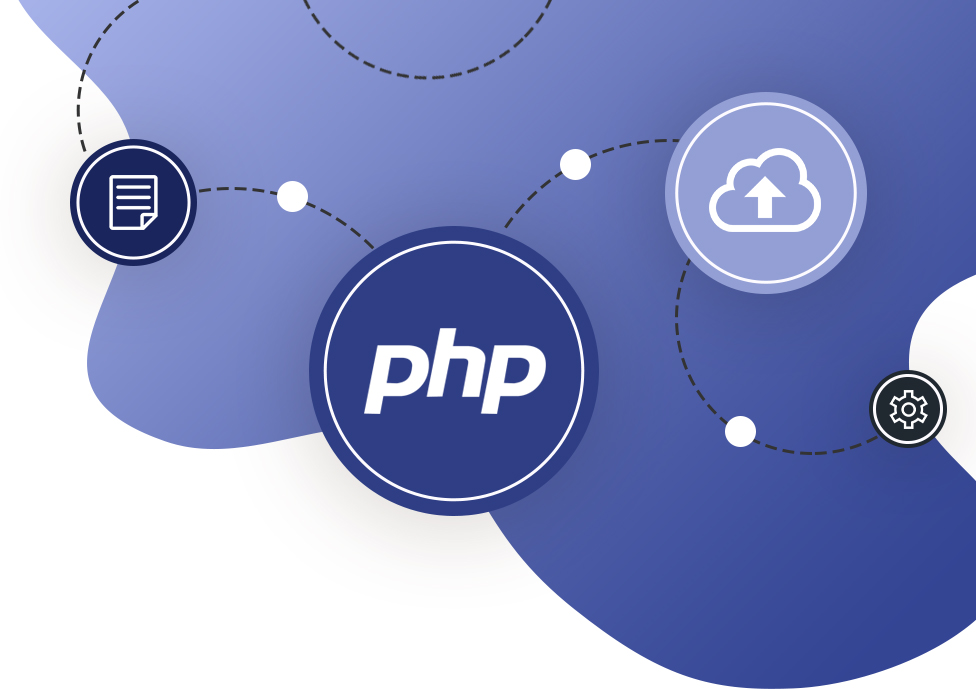
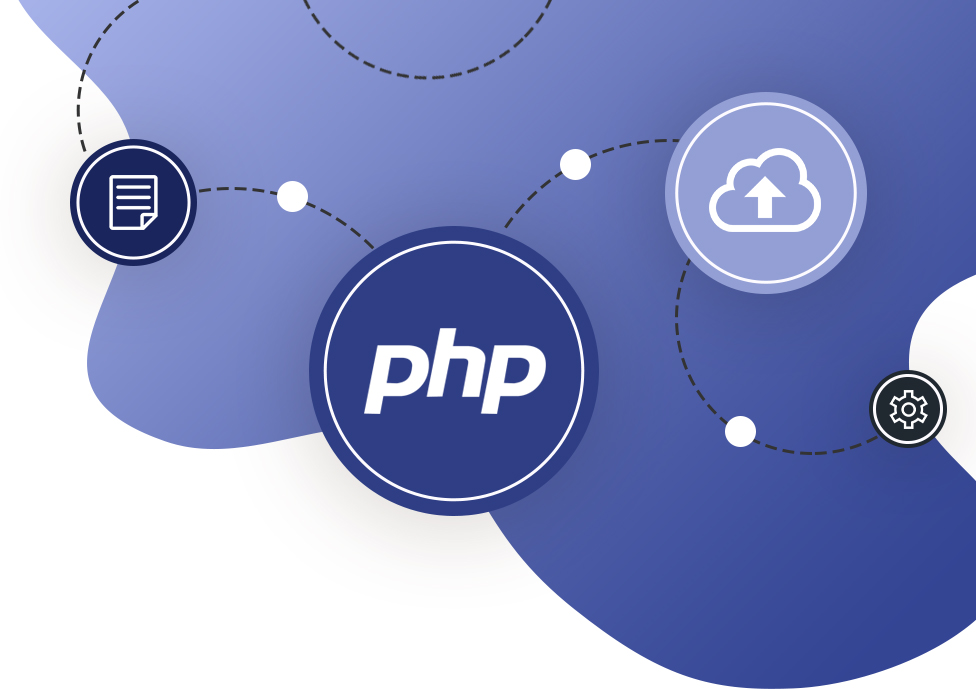
Functions that work with strings in PHP
- 28-07-2022
- Toanngo92
- 0 Comments
PHP supports built-in functions for working with strings. In essence, if the functions are not provided or the situations are complicated, we can use algorithms to handle it, but with the help of built-in functions, it helps us to reduce algorithm writing time, do not need to care about how the math function behaves and only need to care about the input and output of the function.
Full reference here: https://www.php.net/manual/en/ref.strings.php
Some basic string functions:
strlen() : Returns the length of the string. strlen() is a built-in function that calculates the length of a string, including all special characters and spaces.
Syntax:
strlen(string)
For example:
<?php echo strlen("Hello Toan!"); // output: 11 ?>
str_word_count() : The str_word_count() function returns the number of words in a string.
Syntax:
str_word_count(string, return_value, chars)
In there:
string: is the input string.
return_value: specifies the retum value of the function and can be one of the following: 0 (returns the number of words found), 1 (returns an array containing all the words found in the string) or 2 ( returns an associative array) (optional)
chars: specifies a list of additional characters to be treated as a word (optional)
For example:
<?php echo str_word_count("Hello Toan"); //output: 2 ?>
strrev() : This is a predefined function used to reverse a string. This is one of the most basic string operations that programmers and developers use.
Syntax:
string strrev(string $string );
For example:
<?php echo strrev("Hello"); // output: olleH ?>
strpos() : This function is used to find a specified text in a string. If there is a match, it returns the character position of the first matching value that is an integer; if none, it returns FALSE .
Syntax:
strpos($string, $find, $start) ;
For example:
<?php echo strpos("Hello Toan!", "Toan"); // output: 6 ?>
str_replace() : Function used to replace characters in a string.
Syntax:
str_replace($search, $replace, $string, $count)
In there:
- $search indicates the value being searched
- $replace is the replacement value
- $string is the string to replace
- $count indicates the number of successful replacements
For example:
<?php echo str replace("Toan", "Hello", "Hello Toan!"); //output: Hello Hello! ?>
ucwords() : This function returns a string after converting the first character of each word in the given string to uppercase.
Syntax:
string ucwords(string $str)
For example:
<?php $str="my name is toan."; $str_ucwords($str); // output: My Name Is Toan ?>