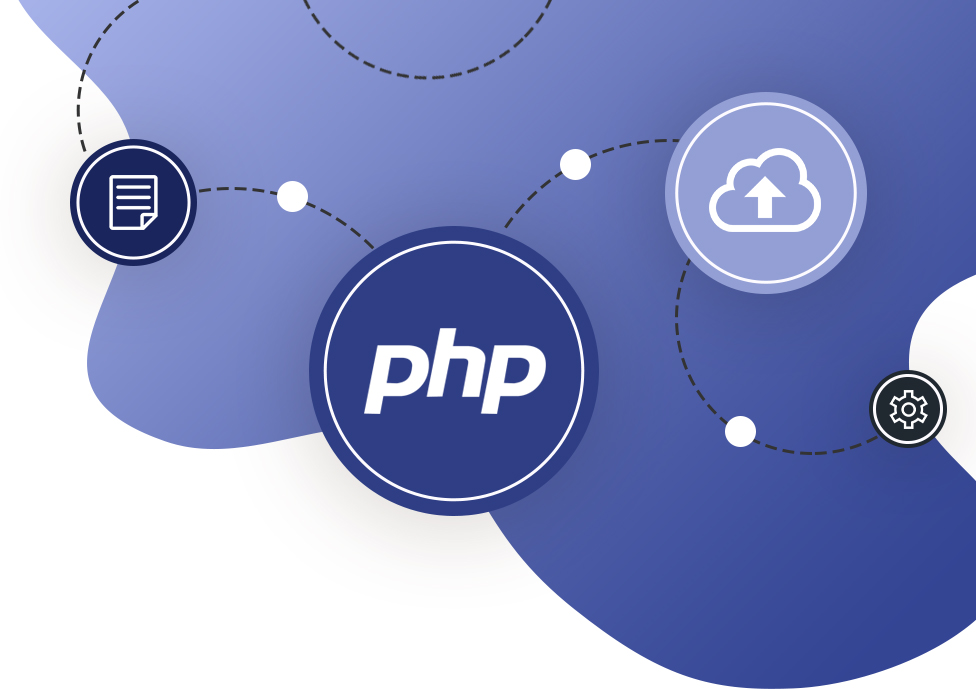
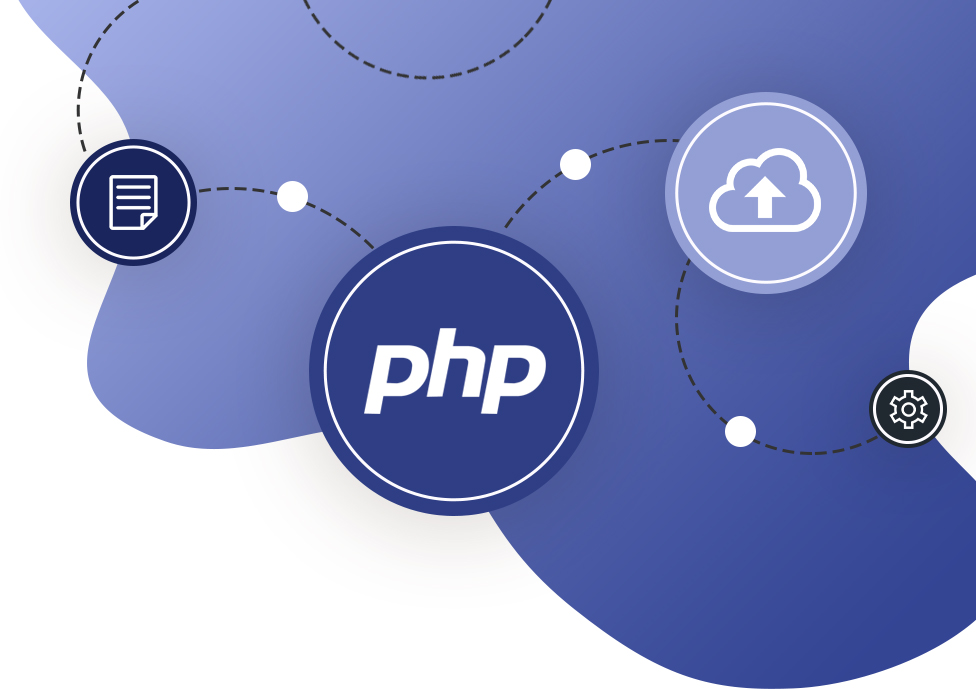
Installing PHP and introducing PHP basics
- 25-07-2022
- Toanngo92
- 0 Comments
Mục lục
Install PHP
System requirements to run PHP
Users must install the following three pieces of software on their local machine to run PHP programs:
- Web Servers like Apache
- PHP interpreter
- Database like MySQL.
Of these 3, Database is optional, however it is recommended if one is creating real applications and PHP almost always comes with MySQL.
Users can separately install a Web server ( Web Server ), a PHP Interpreter ( PHP Interpreter ) and MySQL database through downloading from the respective Official Websites. However, open source developers have created all-in-one setup packages like WAMP, LAMP, MAMP or XAMPP to handle these settings with ease. The specific package to choose depends on the platform the user is comfortable with. Such a package will set up a PHP environment on the user’s Windows, Linux or Mac computer.
All three ( LAMP , WAMP and XAMPP ) have common components: Apache HTTP Server, MySQL and PHP .
The Apache HTTP Server is the most important part of the respective package. It runs an open source Web server on Windows or Linux . When the Apache Web server is running on a local Windows / Linux machine, a developer can test local Web pages in a browser. This effectively means that the Web developer may not have to publish and make this page visible to the Internet just for testing.
Actually, besides Apache, we can also use a few other http servers like nginx, litespeed to run php. However, from a learning perspective, the Apache approach is enough to start learning.
MySQL and PHP are two other components of the respective packages. These two technologies are the most commonly used to create Dynamic Websites.
PHP is a scripting language that can be used to access data from a high-speed MySQL database . Although PHP , MySQL , and Apache are open source components that can be installed individually, they are often installed together for faster productivity.
LAMP
LAMP stands for Linux, Apache, MySQL and PHP. LAMP is the most commonly used solution stack for various Web applications for Linux.
WAMP
WAMP stands for Windows, Apache, MySQL and PHP. The WAMP equivalent is a variant of LAMP for Windows systems and is usually installed as a software package (Apache, MySQL and PHP). Its main purpose is to facilitate Web development and internal testing. In addition, it can also serve Live Websites by acting as a local Web server.
WAMP can handle dynamic web pages, is easy to use with PHP and is available
in both 32-bit and 64-bit systems.
XAMPP
XAMPP is an open source cross-platform Web server solution package that includes an Apache HTTP Server, a MariaDB database, and a PHP interpreter. Moreover, it is free. MariaDB is a community-developed RDMS that has replaced MySQL.
It’s easy to switch from a local test server to a live server because most Web Server implementations use the same components as XAMPP. XAMPP helps developers create and test their programs on a local web server. It allows developers to deploy LAMP or WAMP more easily and faster on the operating system.
You can see the xampp installation guide to install the localhost environment and start programming PHP. If you want to install PHP manually, see the instructions below.
Install PHP on Windows 8.0 and higher with Apache
Minimum requirements for PHP are at least Windows 2008/Vista, 32-bit or 64-bit. Windows 2008 or Vista is not supported from PHP 7.2.0 onwards.
PHP requires Visual C Runtime (CRT). Since many applications require it, it is most likely already installed.
Most recent PHP versions work perfectly with Microsoft Visual C++ Redistributable for Visual Studio 2019. User must download x64 CRT for PHP x64 Builds and x86 CRT for PHP x86 Builds.
If the user is using Internet Information Services (IIS) but wants to set up PHP, the simplest technique is to use Microsoft’s Web Platform Installer (WebPI).
See also how to install PHP manually on different environments:
- Manual php installation for windows
- Manual php installation for MacOs
- Manual php installation for linux
Basic knowledge of PHP
PHP is popularly used to build dynamic and highly interactive Web pages for a powerful user experience. It also communicates with the database and provides more flexibility and simplicity.
For this framework, it is assumed that XAMPP is installed with PHP 8 and MySQL on Windows systems and that all code will be run in this environment.
How PHP works
When a user navigates to the .php page from their Web browser, the browser sends an HTTP request to the Web server. For example, when the user types the URL of the file index.php in the browser and presses Enter, the browser will send a request to the Web server and the server will start looking for this file on its file system. If the Web server locates the file, it sends it to the PHP interpreter.
Otherwise, the Web server will generate a 404 Error or File Not Found.
The Web server only sends files with the .php extension to the interpreter. Other files with extensions like .html, .htm, etc. will not be sent to the PHP interpreter, even if they contain PHP code inside.
When the file is sent to the PHP interpreter, it scans through all the opening and closing PHP tags and then proceeds to process the PHP code in these tags.
PHP interpreter additionally checks if there is a database connection, If a database connection is detected, it sends or retrieves data from the database after proper authentication.
The PHP scripts are interpreted on the Web server and the results (HTML) are sent back to the client.
Writing PHP Scripts
Users can use a text editor to write PHP code. There are many good editors out there that offer strong language support and features like autocomplete, syntax highlighting, code prompts, etc. Notepad++, Sublime Text and Visual Studio Code, PHP Storm is one of the suitable options.
The basic structure of a PHP script mainly consists of:
- PHP opening and closing tags
- PHP code combined with HTML markup
- Comment in PHP (optional)
PHP Tags (PHP tags)
A PHP block begins with the tag “<?php” and closes with the tag “?>”
For example:
<?php echo "I'm Toanngo92"; // to do here ?>
PHP combined with HTML
PHP is designed to work with HTML, so users can easily write and embed PHP inside HTML and vice versa.
For example:
<?php // khai bao bien ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello</title> </head> <body> <h1><?php echo 'Kết hợp php cùng thẻ h1'; ?></h1> <?php echo '<h2>Kết hợp php cùng thẻ h1</h2>'; ?> </body> </html>
In the above example, PHP’s echo command is used, which allows the user to write output data to the browser. Each PHP statement ends with a “;” (semi-colon). In case you write another statement without completing the first statement with a semicolon, PHP will throw a syntax error.
Execute PHP Script
To run or execute a PHP program, the user must save the code to the Web server in the www or htdocs directory (depending on how the installation is done) with the extension .php , Once done, the server must be booted to execute.
Assume that XAMPP is installed with PHP 8.0.13. XAMPP will create an htdocs directory in which PHP scripts can be placed.
Once the server is up and running, the user has to open a Web browser, navigate to localhost and enter the path of the file, for example: http://localhost/test.php
For example, the output of the above Code Snippet. The file is saved under the path C:xampphtdocstest.php but in the browser it should be launched as http://localhost/test.php
Users can also run or execute a PHP script on a black screen (terminal/command line) without
HTML tags.
Steps to run PHP script in command line:
Make sure the PHP environment variable installation path is included in the system and points to the correct php executable directory (such as C:php ), or (C:xamppphp) if you use xampp to be accessible from the command line.
To add a path, go to Control Panel . Then click on Advanced system setting and open Environment Variables . Click on System Variables and then, select PATH and add the path as shown, then click OK, restart the machine to make sure the environment variable is loaded.
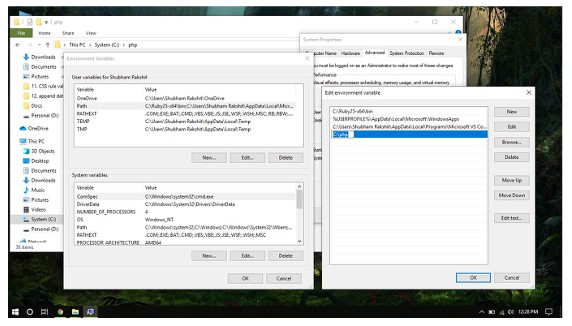
To test whether the environment variable has been added successfully, you can open a command line window and type the following command:
php -v
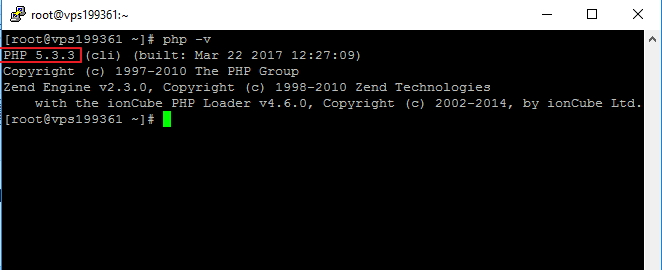
Once the Path is set, the php.exe command can be executed at the command line from any directory, not necessarily the directory where PHP is installed.
For example, create a file program1.php and save it to the php_project folder in drive D and the file content
<?php echo "Hello i'm Toanngo92" ?>
Run the command and output:
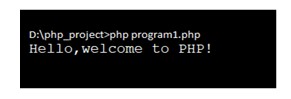
Comments in PHP
Writing comments in a program is essential in practice, as it makes the code easy to read as well as understandable for developers. Consider that a developer Mark Curly works for a company that has written substantial code for several product applications. Now, Mark Curly has left his job and his code has been assigned to another developer, Toanngo92 to continue to maintain and develop more source code. However, Peter finds it confusing and cumbersome to understand what Mark Curly is doing in the code because there is no documentation and no comments. If Mark Curly uses the right comments in his code, then Peter will be a seamless process to take over the source code.
Therefore, comments play an important role in documenting the source code.
The PHP interpreter ignores the execution of comments blocks, thus making the source code readable without impacting performance. Thus, comments can be used anywhere in the program to add information about blocks of code.
In PHP, // or # can be used to create a single-line comment and /* with */ to create a large multi-line comment block.
For example:
<?php // khai bao bien ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello</title> </head> <body> <?php // comment mot dong /* comment nhieu dong comment nhieu dong comment nhieu dong */ ?> </body> </html>