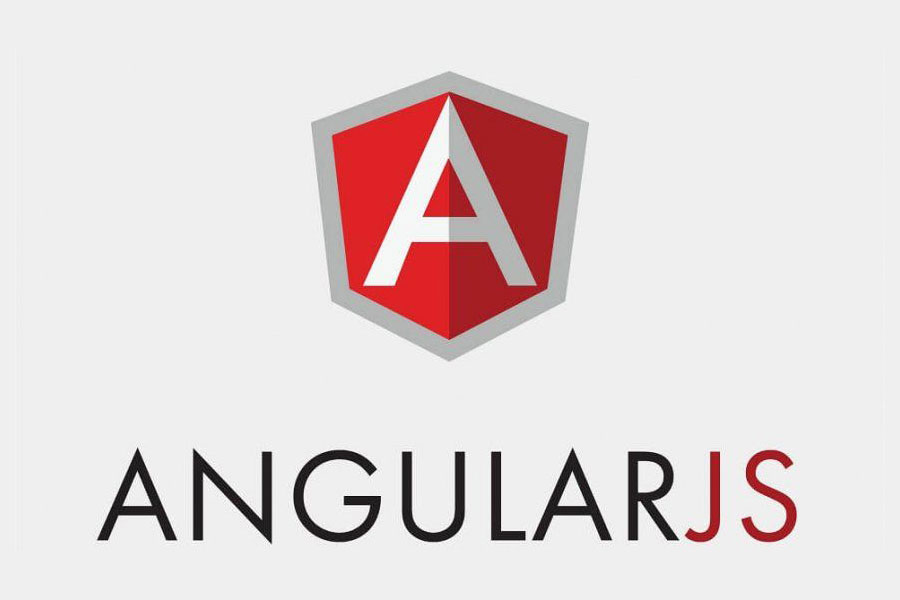
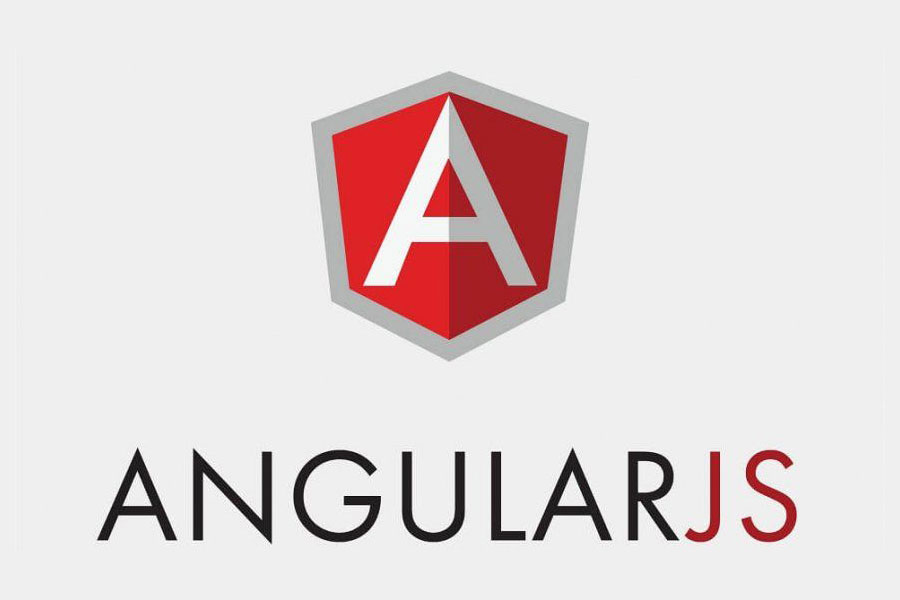
Integrate AngularJS into the project and write hello world application
- 24-07-2022
- Toanngo92
- 0 Comments
In this article, we will start a Hello world program with angularJS
Mục lục
Download AngualarJS
You go to the homepage of AngularJS, click DOWNLOAD ANGULARJS and download the framework:
Visit: https://angularjs.org and download the AngularJS framework:
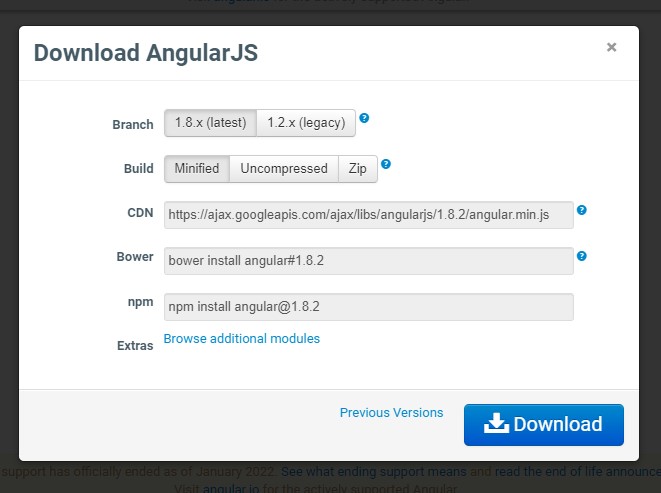
Run environment settings (optional)
To get started with Angular, it is completely possible to put in your HTML project directory and run it, however, if used with this solution, when using the template in angular will get an error, so it is recommended to install server local host environment such as XAMPP or NodeJS as the runtime environment. You can see the article on how to install localhost using xampp
Start writing Helloworld program
Because to get started with angularJS we need a combination of model, view, controller, along with framework properties, for that reason before understanding the problem, we run this sample source code first and then Learn more about the problem in the following articles.
Step 1: Create a project directory, initialize the index.html file and import the Angular library as follows (with the way below I use CDN):
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello world angular</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body> </body> </html>
Step 2: Start the code structure as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello world angular</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body> <div ng-app="toanngo92App"> <div ng-controller="toanngo92Controller"> <h1>Hello {{name}}</h1> <p>You are {{age}} years old, your score is {{score}}</p> <input type="text" ng-model="name"> </div> </div> <script> var app = angular.module('toanngo92App', []); app.controller('toanngo92Controller', function($scope) { $scope.name = 'Toanngo92'; $scope.age = 18; $scope.score = 9.5; }); </script> </body> </html>
Results when running the code in the browser:
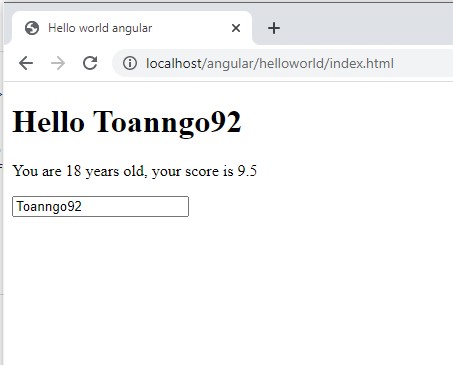
Analyze the code just written in sequence:
- Integrate Angular framework into the project on line 8
- Line 11: <div ng-app="Toanngo92App"> Informs angular that the element(s) fragment(tags) Angular can understand and communicate through the ng-app attribute, the value "toanngo92App" is the name of the application, can be set arbitrarily. When using attribute in HTML structure, it makes sense that only elements from the tag containing the attribute to the elements inside can be queried by angular. Take note of this!
- Line 19: initialize angular object via function angular.module('toanngo92App',[]), with the first parameter being the application name, the next parameter is the empty array, which will be mentioned more in the following articles. .
- Line 20: call controller method, inside are 2 parameters, first parameter is a string defining controller name 'toanngo92Controller', second parameter is an anonymous function with parameter being a builtin variable ($scope), inside this function, define 3 properties for $scope variable name,age,score, this is model
- Lines 12 – 16: inside the ng-app tag structure, is a tag structure with the attribute ng-controller="toanngo92Controller", here we have the understanding that this is the view returned from the controller named " tonngo92Controller" , the data in the controller will pass through the view through the $scope . variable
Next, let's try to enter more data into the input box displaying tonngo92 data, we will see that the data on the h1 tag after the word hello is updated according to the entered data without writing any additional lines of js code, this is the data-binding mechanism and the power of angularJS, with pure javascript or Jquery, the sequential steps would have to be: catch the user's onkeyup event, get the data from the input in focus, then update it on <h1> tag.
Here is an example code of a simple billing application with angularJS:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Hello world angular</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script> </head> <body> <div ng-app="calculateApp" ng-controller="calculateController"> <label>Quantity:</label> <input type="number" min="1" max="10" ng-model="qty" /> <label>Price</label> <input type="number" min="0" max="5000" ng-model="price" /> <div>Total: {{total | currency: '$ '}}</div> <button ng-click="calculate()">Calculate</button> </div> <script> var app = angular.module('calculateApp', []); app.controller('calculateController',function($scope){ $scope.qty = 0; $scope.price = 0; $scope.total = 0; $scope.calculate = function(){ this.total = this.qty * this.price; } }); </script> </body> </html>
Result when running test on browser:
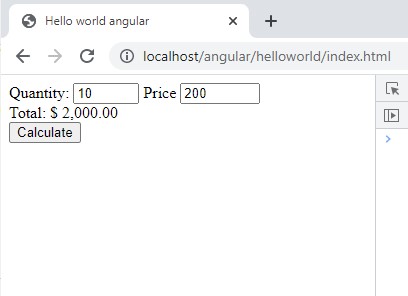
In the above example is a combination of some concepts of angularJS including:
- ng-app, ng-controller,ng-model,ng-click inside the html structure are tag attributes, recognized and used by angularJS, called directives
- {{total | currency: '$ '}} This concept is called default filters of angularJS (refiltering the value for the model when displayed)
- $scope is angularjs builtin variable, used to communicate common data between controller and view
- angular.module() , app.controller() , these are the built-in methods in the angularJS structure that will be approached in the following articles
For this article, write your own first helloworld program, before diving into the theoretical concepts in later articles.