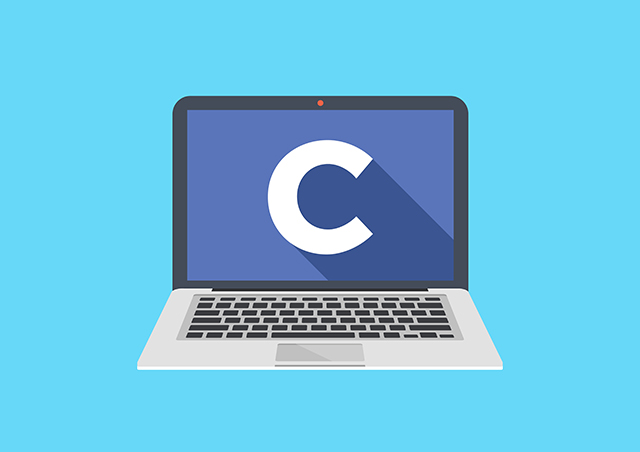
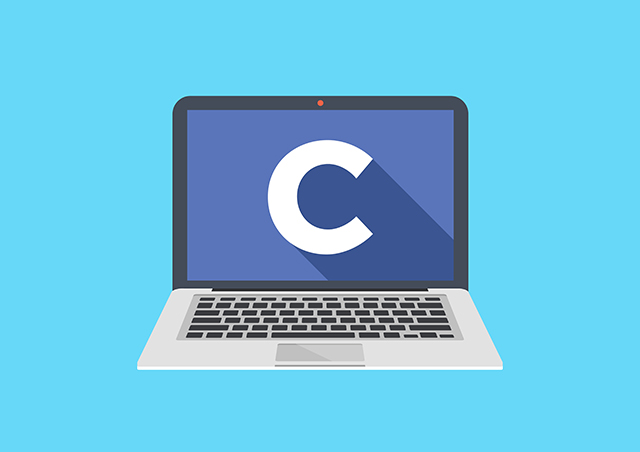
C program structure, list of keywords (keywords) in C language
- 24-07-2022
- Toanngo92
- 0 Comments
The C programming language is a case-sensitive programming language, where we combine pre-existing keywords, usually abbreviations of common English words and constructs known by the language. c defines, accompanied by character sets, to instruct the computer to execute the program
Mục lục
Set of valid alphabets, numbers, or special characters in the C language.
Alphabets (AZ includes both uppercase and lowercase letters)
Uppercase: AZ Lowercase: az
Number (number)
0 1 2 3 4 5 6 7 8 9
Special Characters
, . _ ( ) ; $ : % [ ] # ? ' & { } " ^ ! * / | - ~ +
Keyword table in C
Keywords are words that are predefined by the C language, have clear semantics and are used for specific purposes. You can't use it as a resource (variables, constants – will be discussed in the following articles), so you have to keep it in mind to avoid situations where you declare duplicate content with keywords that cause confusion for the program. (computers can't understand). There are 32 keywords in the C programming language:
auto break case char const continue default do int long register return short signed sizeof static struct switch typeof union unsigned void volatile while double else enum extern float for goto if
Note: All keywords in C are written in lowercase.
The basic meaning of the keywords:
auto, signed, const, extern, register, unsigned – Use in case of resource declaration (variable).
return – The keyword is used to (program, function) to return a value.
continue – Usually used with for, while, do-while loops, when this statement is encountered in the body of the loop, the program will switch to a new loop and skip the execution of the following statements. this command in the loop body.
enum – user-defined data type. Commonly used to assign names to constants, making a program easier to read, code cleaner, and maintain.
sizeof – Returns the size (bytes) of the memory area.
struct , typedef – Both of these keywords are used in structs.
union – Allows creating different data types in the same memory area.
volatile – Avoid unexpected errors when the compiler's optimization feature is enabled. Volatile refers to a variable that can be changed in an "abnormal" way (often used in embedded programming).
Program structure C
command concept
In programming languages, we use instructions to perform computer program processing operations, a program will consist of a set of many different instructions. The C programming language doesn't care about line breaks or the spacing of elements inside a statement. Each statement is separated by ";". For example
int i; i = 5; // cú pháp vẫn đúng int a a = 6; // sai vì câu lệnh khai báo chưa có kết thúc bằng dấu ;
Function concept
A function is a group of statements that perform the same task . Every C program has at least one function, the main() function, and all programs can define additional functions.
consider the following code:
#include <stdio.h> #include <stdlib.h> int main(void){ return 0; }
In this code, we are declaring a function named main() , and this is a special function, when the C program starts running, the main function will always be found and executed. First of all, that means, no matter how long your code is, how many functions there are, what the source code is, the main function will always be found by the operating system and given the right to execute as soon as the program starts. running head. The function name is always followed by a single parenthesis (), in the parentheses there may or may not be a parameter ( parameter )
Delimiters
Go back to the code above and observe, after () we see a pair of curly brackets {}, this is a marker for the beginning of the function declaration, understood as the description. a set of tasks to be executed, and these tasks are encapsulated in a descriptive name, the function declaration is to describe the tasks to be executed so that the computer understands and when we need to execute them, we just need to name that task, the jobs inside will be executed one after another until the signal ends (the opening bracket "{" is the start of the tasks, the closing parenthesis " }" signals the end of tasks)
Comments (Comments)
This concept you will see very often in most other programming languages, if we want to annotate the meaning of a piece of code, or annotate something in the code, use this concept. The syntax of comments in C programming is as follows:
// comment inline /* comments multiple lines comments multiple lines comments multiple lines */
C library (Library)
The C compiler (understanding as a dictionary that helps to compile the code we write into a language that computers can understand and execute) contains a lot of standard functions, predefined code snippets, and many more. can be used in general, even for further development, when programming, we use the library as a solution to be able to get the functions and definitions already available for application development without needing to go through the process. caring what the bottom layer is, is as simple as saying to a computer: hey, print me this text. The computer will do it for you and print the text, the computer has already defined it in the dictionary, you just jump into the dictionary and read the word up for the computer to follow.
The process of compiling and executing source code in C

1.Preprocessing
The C program (source code) is sent to the preprocessor first. The preprocessor is responsible for converting the preprocessor directives into their respective values. The preprocessor generates an extensible source code.
2. Convert source code into assembly language
Extensible source code is sent to the compiler to compile the code and convert it into assembly.
3) Turn source code into object
Assembly code is sent to the assembler to assemble the code and convert it into object code. Now a simple.obj file is created.
4) Link to the library and convert to executable code
The object code is sent to the linker that links it to the library, such as header files. It is then converted into executable code. A simple.exe file is created.
5) Executing the program
The executable code sent to the loader loads it into memory and then it is executed. Once executed, the output is sent to the console.
If you've read this far, we can already start getting into the specifics!