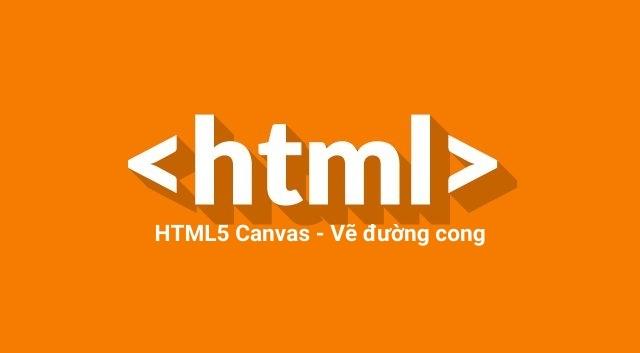
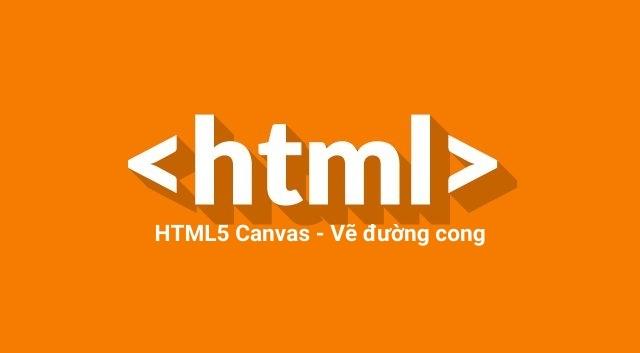
HTML5 Canvas – Draw Curves
- 24-07-2022
- Trung Minh
- 0 Comments
In addition to drawing straight lines, Canvas can also draw curves such as arcs, quadratic curves, and Benzier curves. For how to draw a straight line, we only need to determine the beginning and end points, but with curves, there are quite a few problems to calculate before drawing it.
1. Draw an arc in Canvas
To draw an arc, we use arc(x, y, radius, startAngle, endAngle, counterClockwise)
, where:
-
x
andy
are the centers of the arc -
radius
is the radius -
startAngle
is the starting angle -
endAngle
is the end angle -
counterClockwise
is drawing direction ( clockwise or counterclockwise )
var canvas = document.getElementById('myCanvas'); var context = canvas.getContext('2d'); // Xác đinh tâm là trọng tâm của Canvas var x = canvas.width / 2; var y = canvas.height / 2; // bán kính 75px var radius = 75; // Góc bắt đầu là 1.1PI var startAngle = 1.1 * Math.PI; // Góc kết thúc là 1.9PI var endAngle = 1.9 * Math.PI; // Cùng chiều kim đồng hồ var counterClockwise = false; // Vẽ đường cung context.beginPath(); context.arc(x, y, radius, startAngle, endAngle, counterClockwise); context.lineWidth = 15; // line color context.strokeStyle = 'black'; context.stroke();
2. Draw a quadratic curve in Canvas
To draw a quadratic curve in Canvas, we use the quadraticCurveTo()
method, the quadratic curve is determined by three main points including:
- The starting point ( context point ).
- Control point ( control point ).
- End point ( end point ).
var canvas = document.getElementById('myCanvas'); var context = canvas.getContext('2d'); context.beginPath(); context.moveTo(20, 20); // Điểm bắt đầu vẽ context.quadraticCurveTo(20, 100, 200, 20); //vẽ qua các điểm context.lineWidth = 1; // line color context.strokeStyle = 'black'; context.stroke();
- The starting point is ( 20, 20 ) in the
context.moveTo( 20, 20 )
function. - The control point is ( 20, 100 ) in the function
context.quadraticCurveTo( 20, 100 , 200, 20)
. - The end point is ( 200, 20 ) in the
context.quadraticCurveTo(20, 100, 200, 20 )
function.
The vertex position of the curve is determined by the line connecting the midpoints between the two lines ( context point, control point ) and ( end point, control point ).
var canvas = document.getElementById('myCanvas'); var context = canvas.getContext('2d'); context.beginPath(); context.moveTo(188, 150); context.quadraticCurveTo(288, 0, 388, 150); context.lineWidth = 10; // line color context.strokeStyle = 'black'; context.stroke();
3. Draw a Benzier Curve in Canvas
The Benzier curve is defined by four points:
- The starting point ( context point )
- Control point one ( control point 1 )
- Control point two ( control point 2 )
- End point
var c = document.getElementById("myCanvas"); var ctx = c.getContext("2d"); ctx.beginPath(); ctx.moveTo(20, 20); ctx.bezierCurveTo(20, 100, 200, 100, 200, 20); ctx.stroke();
The explanation in the code is as follows:
- The starting point is ( 20, 20 ) in the
context.moveTo( 20, 20 )
function. - Control point 1 is ( 20, 100 ) in the function
context.quadraticCurveTo( 20, 100 , 200, 100, 200, 20)
. - Control point 2 is ( 200, 100 ) in the function
context.quadraticCurveTo(20, 100, 200, 100 , 200, 20)
. - The end point is ( 200, 20 ) in the
context.quadraticCurveTo(20, 100, 200, 100, 200, 20 )
function.
The question is how to determine the vertex and curvature? See the example below.
var canvas = document.getElementById('myCanvas');<br /> var context = canvas.getContext('2d');<br /> <br /> context.beginPath();<br /> context.moveTo(188, 130);<br /> context.bezierCurveTo(140, 10, 388, 10, 388, 170);<br /> context.lineWidth = 10;<br /> <br /> // line color<br /> context.strokeStyle = 'black';<br /> context.stroke();
4. Conclusion
Starting to find it difficult to work with Canvas already. Really, to draw good images, you have to calculate each standard Pixel, not to mention having to use math knowledge to determine coordinates.
In the next lesson we will learn how to draw a line using the functions learned in this and previous lessons.