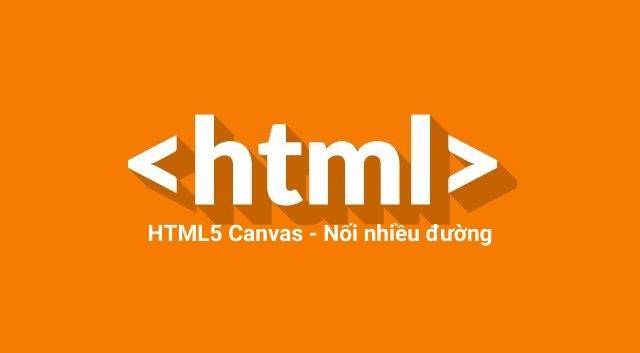
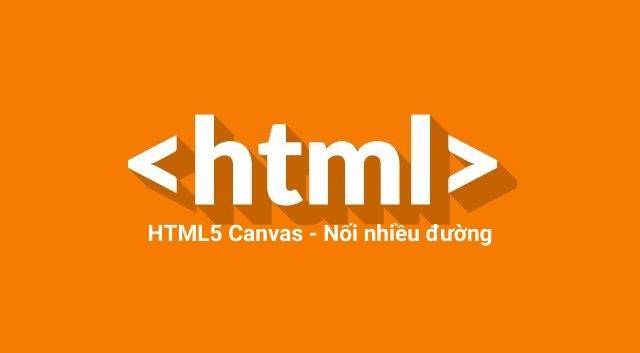
HTML5 Canvas – Join multiple lines
- 24-07-2022
- Trung Minh
- 0 Comments
We have already learned how to draw some lines in Canvas, so in this article I will show you how to connect those lines together to create much more vivid drawings.
1. Connect multiple lines together in Canvas
As we know in Canvas we use beginPath()
function to declare the start of drawing a new shape. So to connect multiple lines together, we only declare it true once first, then rely on the syntax of each line to determine the drawing points.
Example : Draw a figure like this
We first analyze this figure as follows:
So we will use two lines, a quadratic and a Benzier line.
var canvas = document.getElementById('myCanvas'); var context = canvas.getContext('2d'); context.beginPath(); context.moveTo(100, 20); // line 1 context.lineTo(200, 160); // quadratic curve context.quadraticCurveTo(230, 200, 250, 120); // bezier curve context.bezierCurveTo(290, -40, 300, 200, 400, 150); // line 2 context.lineTo(500, 90); context.lineWidth = 5; context.strokeStyle = 'blue'; context.stroke();
2. Line join property in Canvas
In Canvas, there is a lineJoin property that helps us choose how to join two lines ( intersection ). It has three ways to connect as follows:
- miter : to make an acute angle
- round : rounded corners
- bevel : rounded to a sharp corner but lost the top part
var canvas = document.getElementById('myCanvas'); var context = canvas.getContext('2d'); // set line width for all lines context.lineWidth = 25; context.strokeStyle = 'blue'; // miter line join (left) context.beginPath(); context.moveTo(99, 150); context.lineTo(149, 50); context.lineTo(199, 150); context.lineJoin = 'miter'; context.stroke(); // round line join (middle) context.beginPath(); context.moveTo(239, 150); context.lineTo(289, 50); context.lineTo(339, 150); context.lineJoin = 'round'; context.stroke(); // bevel line join (right) context.beginPath(); context.moveTo(379, 150); context.lineTo(429, 50); context.lineTo(479, 150); context.lineJoin = 'bevel'; context.stroke();
3. ArcTo() function in Canvas
The arcTo() function is used to create an arc in Canvas with a given starting point, that is, it is used to connect a certain line ( of course that line already has an end point and that point is also the point). start of arcTo()
).
Syntax : context.arcTo(x1, y1, x2, y2, r);
In which :
-
x1
: x position of control point -
y1
: y position of the control point -
x2
: x position of the end point -
y2
: y position of the end point -
r
: radius of the arc ( radius ).
var c = document.getElementById("myCanvas"); var ctx = c.getContext("2d"); ctx.beginPath(); // Vẽ 1 đường thẳng ctx.moveTo(20, 20); ctx.lineTo(100, 20); // Nôi tiếp là một đường cung ctx.arcTo(150, 20, 150, 70, 50); // Tiếp theo là một đường thẳng ctx.lineTo(150, 120); ctx.stroke(); <br><br><br><br><br><br><br><br><br><br><br><br><br>
4. Conclusion
In this lesson we have learned a few ways to join lines together to form a variety of lines, combining these ways you will create extremely impressive drawings. However, working with Canvas is really difficult because the coordinate calculation is very lengthy and complicated.
The next lesson we will learn how to draw some special shapes called Shapes. For example circle, rectangle, semicircle.