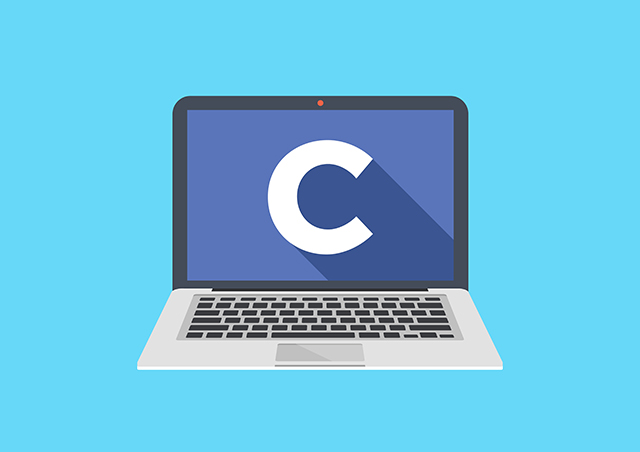
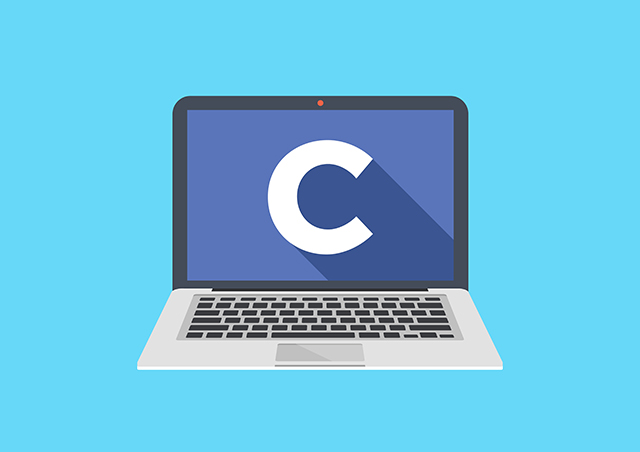
Input / Output Manipulation in C
- 24-07-2022
- Toanngo92
- 0 Comments
Why put the input output creation before the next conceptual lessons? Because to make it easier to practice during the exercise, I will go to input/output and introduce basic input and output first, although we don't understand the concept of a function yet, but let's use it first. , and then go to the following concept.
The C program provides a set of standard libraries to work with I/O functions, input is to put data from the peripheral device, in the context of C programming language is the keyboard, output is to put data from the computer. calculated, this context is to print to the screen.
Mục lục
Output – Print to the screen with the printf . function
In C programming, printf() is one of the output functions that prints data to the main screen. This function is defined in a library named <stdio.h>, to use it we include the standard library according to the syntax
#include stdio.h
For example
#include stdio.h int main() { // in dòng hello world ra màn hình printf("hello world"); return 0; }
The result is printed to the screen:
hello world
Program run flow:
- Include the stdio.h library
- When executing the source code, the C program calls the main function to execute
- In the main function call (execute) the printf function (print the text hello world)
- return 0 to end the program.
Why return 0 at the end of main ? will be covered in the function concept article. In this context, we declare main function with data type int , so at the end the function needs to return 0 to return the value to the operating system and terminate the program. If you don't want to return 0 at the end of the program, declare void data type for main() function.
The printf . function syntax
printf("control string",argument list); // controlstring: chuỗi điều khiển // argument list: danh sách tham số
Format Sepcifier
Consider the example below is the command to print numeric data to the screen
#include int main() { int testInteger = 5; printf("Number = %d", testInteger); return 0; }
Result:
Number = 5
Input – Enter data with the scanf . function
In C language, the scanf function is used to receive data that the user enters from the keyboard. This function will read data from the keyboard input in the correct format that the programmer has a convention similar to the format structure we have approached in the printf function.
scanf . function syntax
scanf("format specifier list",argument list); // format specifier list: chuỗi bộ định dạng // argument list: danh sách tham số
See the example below:
#include int main() { int testInteger; printf("Enter an integer: "); scanf("%d", &testInteger); printf("Number = %d",testInteger); return 0; }
Assuming you enter the number 4, the result will look like this:
Enter an integer: 4 Number = 4
Here, I use the format %d because I want the input data to be an integer.
Example: Float and Double Input/Output
#include int main() { float num1; double num2; printf("Enter a number: "); scanf("%f", &num1); printf("Enter another number: "); scanf("%lf", &num2); printf("num1 = %fn", num1); printf("num2 = %lf", num2); return 0; }
Result:
Enter a number: 12.523 Enter another number: 10.2 num1 = 12.523000 num2 = 10.200000
We use %f and %fl to format floats and doubles.
Example: Character
#include int main() { char chr; printf("Enter a character: "); scanf("%c",&chr); printf("You entered %c.", chr); return 0; }
Result:
Enter a character: g
You entered g
Note: the obvious difference between the printf and scanf functions is the use of the parameter list, with printf we pass the parameter list in with the variable name, and with scanf we need to add the & character before it. variable name, this is the concept of memory location (memory address) will be mentioned in detail in the article pointers. (In that article, I will also explain more why the %s case in scanf can directly pass the variable name as a parameter without using & and still valid)
Import and export multiple data at the same time through the parameter list (argument list)
You can import and export multiple data at the same time by passing more parameters to the printf and scanf functions, and of course the format you need to enter is fully equivalent, matching the order, quantity, and data type. data of the parameter variable.
See the following example, I used the scanf function and the printf function to print two variables at the same time.
#include int main() { int a; float b; printf("Enter integer and then a float: "); // Taking multiple inputs scanf("%d%f", &a, &b); printf("You entered %d and %f", a, b); return 0; }
Result:
Enter integer and then a float: -3 3.4 You entered -3 and 3.400000
Note: when using the scanf function, the formatter strings are written contiguously, without spaces, string constants in between, for example:
scanf("%s %d",&a,&b) // sai scanf("%s va %d",&a,&b) // sai scanf("%s%d",&a,&b) // đúng
Format specifier command table
Format | printf() | scanf() |
Character (char) | %c | %c |
Integer (decimal) | %d | %d |
Real numbers (float) | %f | %f |
Real (double) | %lf | %f |
array character/string (array character/string) | %S | %S |
Decimal with floating point | %e | %e or %f |
Unsigned integer (unsigned) | %u | %u |
Unsigned hexadecimal integer | %x | %x |
Unsigned octal integer | %o | %o |