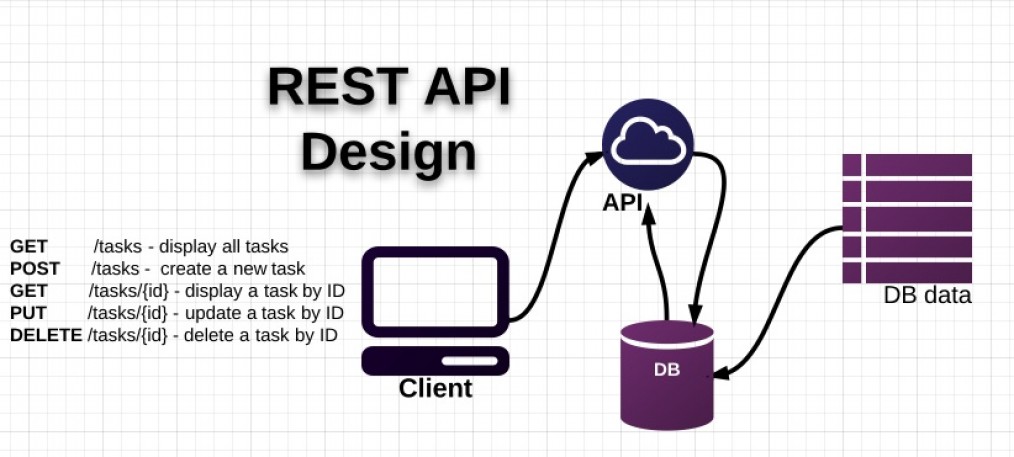
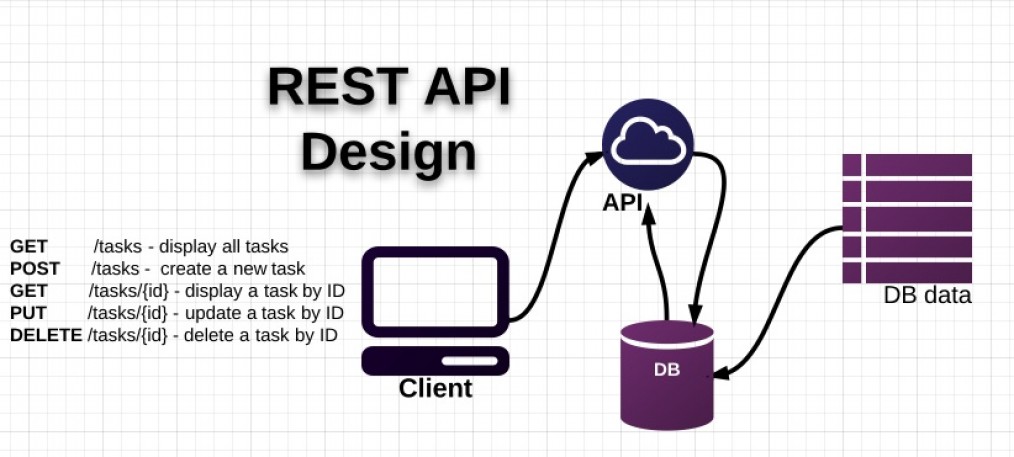
Services communicate and REST API concept
- 25-07-2022
- Toanngo92
- 0 Comments
Mục lục
Introduction to REST API
REST is a stateless architecture , REST is used to build an application that communicates over the network, invented and introduced in 2000, providing a protocol for machine-side communication. client and server. In most cases, this architecture uses a protocol called hypertext Transfer Protocol (HTTP) to communicate between devices.
Before the REST architecture was introduced, programmers used complex communication architectures with basic concepts such as:
- Simple Object Access Protocol (roughly translated as basic object access protocol) is abbreviated as SOAP . See more here
- Remote Procedure Call (remote procedure call) is abbreviated as RPC . See more here
- Common Object Request Broker Archittechture is abbreviated as COBRA . See more here
We temporarily understand REST is a lightweight architecture used for web services. With many different perspectives, WWW (world wide web) based on the http protocol has brought in a REST-based architecture. REST is really optimal for the internet as applications using the http protocol are still popular and powerful. (If you do not understand, imagine when you type the domain name web888.vn on the browser, the browser will automatically add http:// or https:// in front of the domain name string, in addition, some websites add http:/ /www.tenmien.com, this means the browser sets the http protocol as default when accessing the web application)
REST responds to a client-side request on four operations conceptually named Create,Read,Update,Delete (CRUD). A RESTful application sends HTTP requests (requests) that are used to read, add, update, or delete data. In short, REST provides an easy-to-understand architecture so that we can manipulate and customize the data as we want and is provided as a standard library feature in languages like C#, Java, Perl ..
Although this REST architecture is quite comprehensive, in other words, it provides full features, can be called do everything but these things can be done through what is called Web services (web services), REST not recommended by W3C, and not the default mechanism
REST Principles
REST in style is to define a set of constraints used to create a commonly used architecture, these constraints are principles or properties that differentiate REST architectures from other architectures. other
- Client-server communication : basically divided into 2 layers: client and server are 2 separate components, RESTful application ensures they work and helps client (client) to communicate with server (server).
- Statelessnessness : client state information is not stored on the server. In other words, each client-side request will include all the necessary information, the server receives the information and performs the task. It allows the server to understand client-side tasks, the state of each communication session, and the data returned to the client also includes session information for each request (query). Stelessness helps to provide a tight mechanism to communicate between the server and the client to achieve the task purpose
- Cacheability : the client can cache the returned data temporarily, and describe for itself whether the data is cacheable or not, if so, the data will be reused for the next response help increase response time
- Uniform Interface : A standard interface that makes it easy to interact with all the components, which simplifies the interaction with different services. It also helps to ensure that development changes won’t affect other components of the application, on the flip side of being unable to modify the standard.
- Layered system : an intermediary, such as a proxy, that can interrupt communication between the client and the server for a specific purpose such as security, load balancing, or caching. And the client won’t be able to tell if it’s communicating with the server or an intermediary
- Code on demand : this is an arbitrary constraint where the server temporarily extends the client’s functionality by allowing it to download programs, which then execute on the client. For example, a client can run javascript code to interact with another service running on it
Compare REST and SOAP
Many programmers (myself included) ask why REST is more appropriate than SOAP. It’s really difficult for newbies because nowadays, we really rarely have access to SOAP. First, it is practically impossible to compare REST and SOAP directly, because SOAP is a protocol, and REST is an architectural style.
However, the points that help us to evaluate between SOAP and REST are as follows:
Point of difference | SOAP | REST |
Coherence between client and server implementations | Tied to the server, for example a custom computer application, a tight contract, which can be understood as a binding, exists between the client and the server, if one of them change, everything in the process of communication will be broken | A loosely coupled mechanism such as a browser, a REST client is a client application that shares common standardized methods and protocols to which the application adapts. No additional methods are defined to violate protocol standards. changes are handled more easily |
Orientation | Subject | Resources |
Size | Heavy | Light |
Status | Stateful | Stateless |
Standard | Clear data standards | Data standards are not clear |
speed | Slower and consumes more resources and bandwidth per communication | Faster, by consuming less bandwidth and resources |
Communication standards | eXtensible Markup Language (XML) | Use message control to interpret with many modern data structures such as XML, plain text, JSON |
implementation | More complicated (difficult) | Easier |
Client | It is necessary to have a complete understanding of the data architectures in use before interacting | No API knowledge required, except for the entry point and return data type |
Resources in RESTful Web Services
A RESTful Web Service is a communication business (service) in web technology that evolved from the REST API architecture. It has predefined URIs ( Uniform Resource Identifier ) , which refer to the business to instruct HTTP methods.
In RESTful API, all the problems are around the concept (resource) can be translated as resource, we can understand as follows: every time we implement directive api methods, we work with resource, it can be a document, image, file, web page …. A resource has a type (can be visualized as data) containing data inside, and it has a collection of methods. to manipulate it, and possibly have relationships with other resources.
Resource in REST is equivalent to the concept of object in object-oriented programming languages, such as with the problem of student management, adding, editing and deleting classics in C programming language, each variable represents student data structure (including name, age, grade), in modern programming languages with new style syntax, it is an object. And to add and remove them, REST provides some basic methods with CRUD concept for us to approach. However, in practice, we can use more methods, but usually the following 4 methods are just enough and enough to use:
- POST (Create): initialize a new resource or in some situations can also be used to update an existing resource
- GET:(Read) gets a read-only resource, normally used to get an object, or a list of objects.
- PUT:(Update) updates available resources
- DELETE: (Delete) delete resource
Resources (Resource) can be completely grouped into a list, each list is also a resource (Resource), can be understood as such, this list may not be sorted and has uniformity, standard data structure and we can use that data for further processing in programming languages such as parsing into arrays, normalizing to primitive data types, displaying user interfaces .. ..
A REST server allows access to resources and the client represents them, each resource has a unique identifier based on the standard URI (usually a parameter, slug – an element of the transmission path). on the path). For example, an api endpoint as shown to represent an endpoint working with a resource to manage tasks (can be understood as an object):
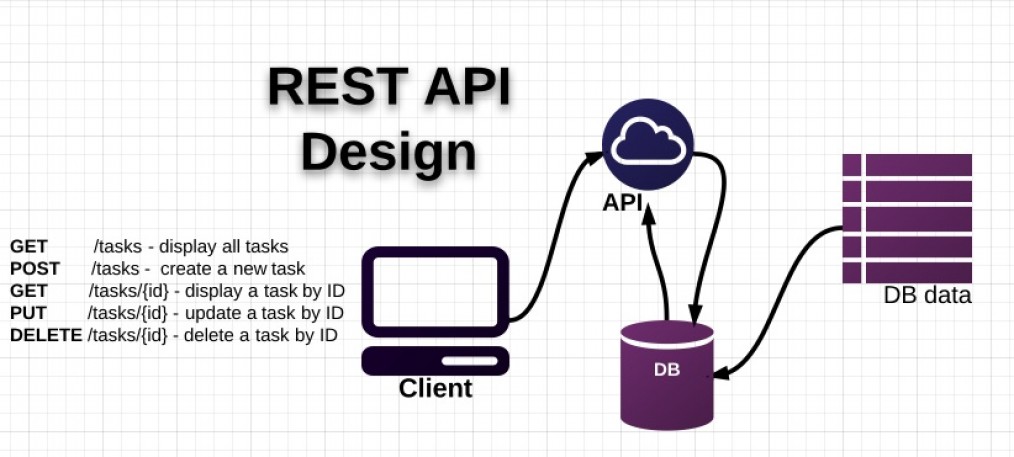
For resource representation, REST supports a variety of data structures from strict (XML) to modern (JSON), or just plain text (PLAINTEXT). Currently and for the foreseeable future JSON is still the most popular when using web services.
HTTP Messages
We can understand HTTP Messages as response messages when communicating with HTTP, RESTful Web service relies on HTTP to initiate communications or issue messages when the client communicates with the server. While processing the message, the client sends an HTTP Request to the server, the server returns to the client a packet called HTTP Response, the request and response message contains the message content (message) with information about the data. data, called metadata. The structure is as follows:
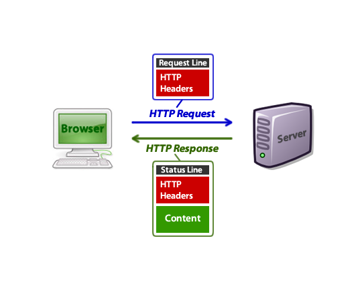
HTTP Request Structure
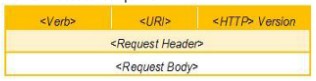
- Verb : represents HTTP methods such as GET,DELETE,PUT,POST
- URI : can be roughly understood as the destination path, identifying the desired resource to work on the server
- HTTP Version : represents the version of HTTP (e.g. v1.1)
- Request Header : contains the file’s metadata, with standard key-value pairs. For example, metadata adds to the request browser information, cache configuration, and format for the message body.
- Request Body : contains the message data in the appropriate format
HTTP Response Structure
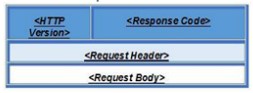
- HTTP Version : represents the version of HTTP (e.g. v1.1)
- Respon code : 3 alphanumeric characters indicating the status of the requested resource. For example 200 is ok, 404 is resource not found
- Response Header : has metadata with response message with key-value pairs, For example metadata tag can include content length, response date, server type, content type
- Response Body : contains the file data with the appropriate format
Example image of request header,
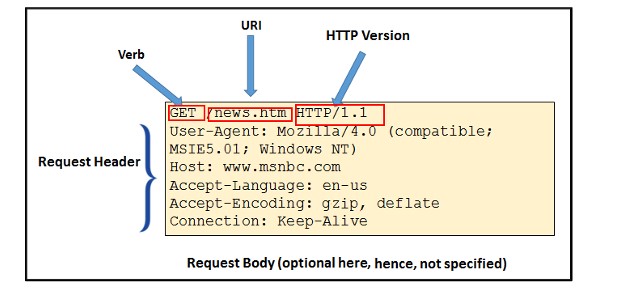
Response Code | Notify | Describe |
200 | OK | Successful |
201 | Created | Indicates that the desired resource has been successfully created or updated via the PUT or POST method, and returns a connection to it via the location header. |
204 | No Content | Appears when the response body is white. For example, this code will be displayed when the DELETE request is executed successfully |
304 | Not Modified | Use for reduced bandwidth usage if there are conditional GET requests, empty response body and headers with metadata like location and date |
400 | Bad Request | Indicates that the client-side input has invalid data given in the request. For example, it may have missing data or inappropriate parameters |
401 | Unauthorized | Indicates that the client is not authorized to access (unauthenticated) |
403 | Forbidden | Indicates that the client was clicked to execute the method, for example, the codes displayed when the client does not have admin rights but wants to perform a data DELETE request on the server resource. |
404 | Not found | Indicates that the method was not found |
409 | Conflict | Indicates that a conflict was encountered while executing the requested method. For example, this code is displayed if the client tries to add a resource with , but the resource already exists. |
500 | Internal Server Error | Indicates that the server caught an exception during method execution |
Software used to access
Client
Postman software can be used as a client when accessing the REST API
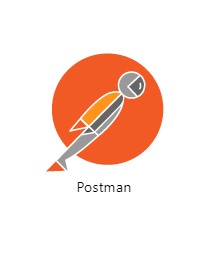
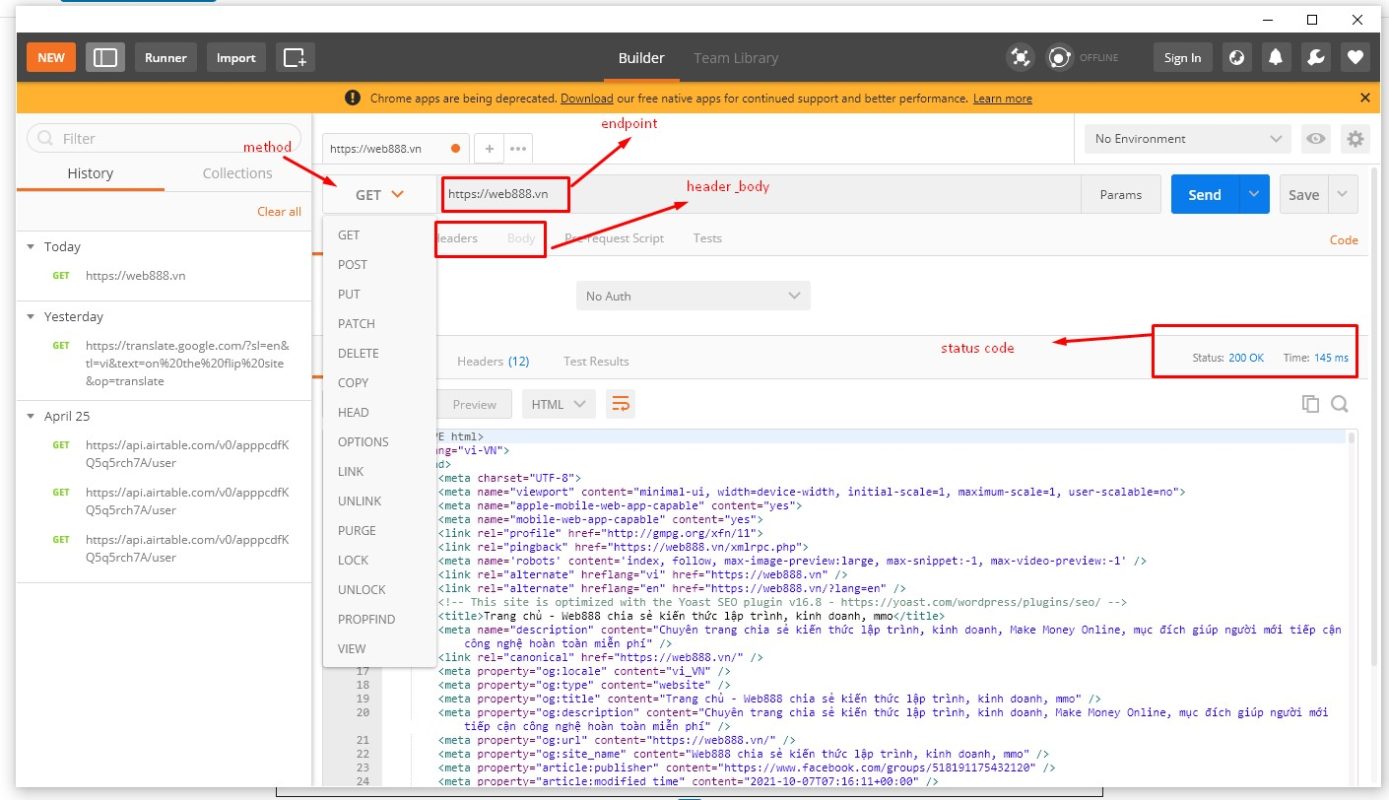
Server
With the server, if you use frameworks for coding, there will certainly be a mechanism to build an API for the server according to the framework structure, if you have not learned, you can approach some of the companies that provide popular APIs to practice client communication, for example:
- Airtable : a row-and-column database storage system with similar features to excel but more developed, providing APIs to communicate more, edit, delete
- Mapbox API : Free Map provides API to be able to draw maps online
- Openweather : provides API to get weather
- …
The above article gives basic concepts when using REST API to interact between client and server, hope after reading you will better understand the concept of architecture as well as how to use REST API. you succeed !