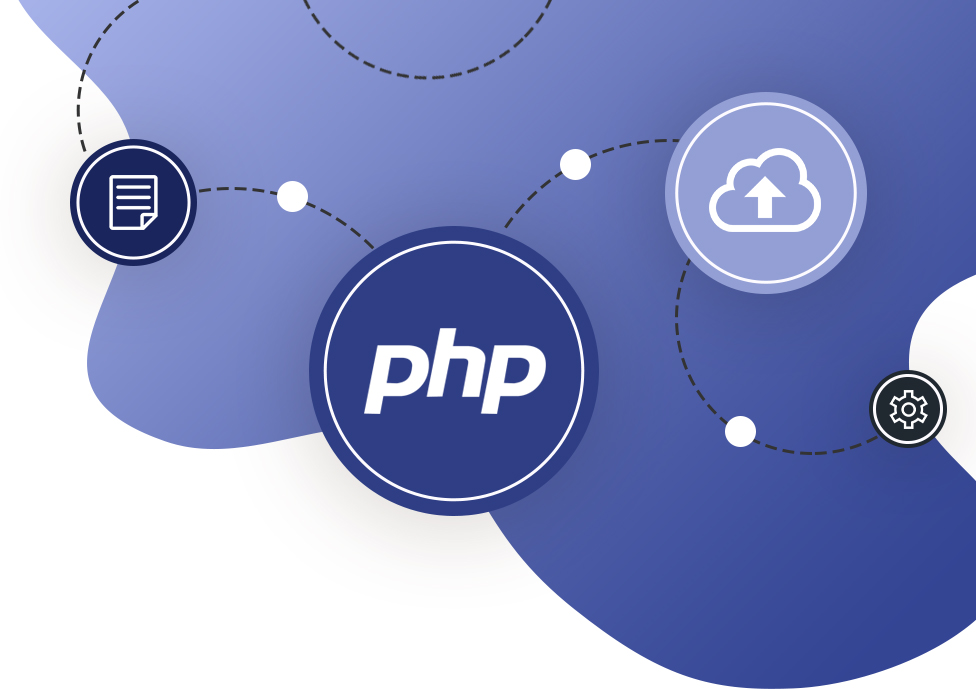
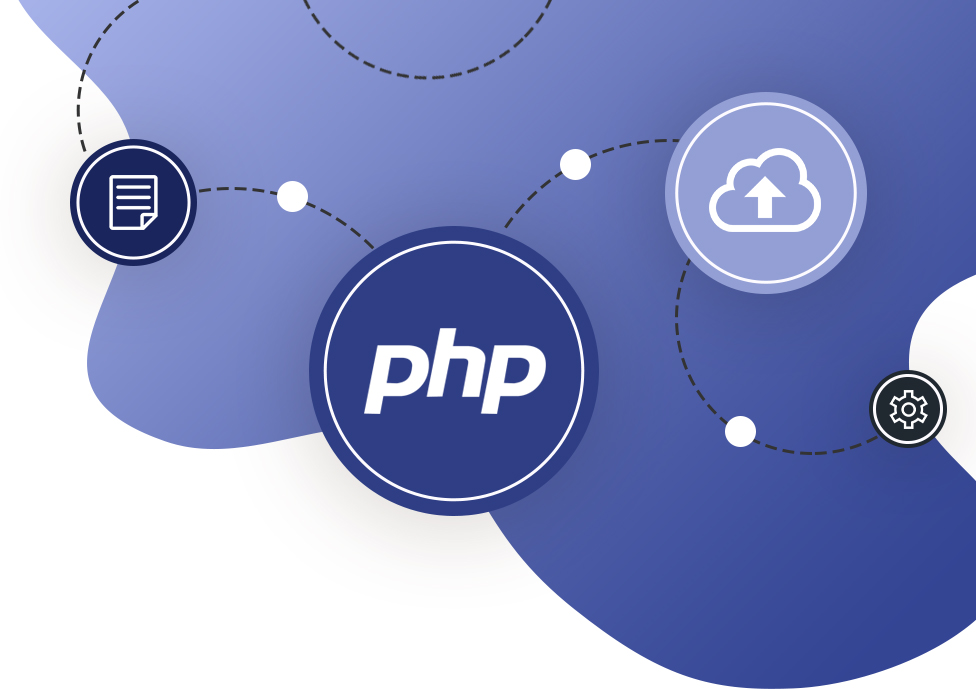
Hằng số trong PHP và cách khai báo hằng số
- 13-07-2022
- Toanngo92
- 0 Comments
Mục lục
Khái niệm hằng số
Hằng số là một thuật ngữ xác định một giá trị duy nhất. Giá trị của hằng số không thể thay đổi trong khi tập lệnh đang chạy. Tên hằng chỉ có thể bắt đầu bằng một chữ cái hoặc một dấu gạch dưới (dấu $ không được đặt trước tên hằng).
Các hằng số, không giống như các biến, tự động là toàn cục trong toàn bộ tập lệnh. Tóm lại, hằng số tương tự biến tuy nhiên một khi đã chỉ định thì không thay đổi được
Định nghĩa hằng số
Cú pháp Khởi tạo hằng số trong PHP:
define(name, value, case-insensitive)
Ví dụ:
<?php
define('VERSION','8.0');
echo VERSION;
// output: 8.0
?>
Hằng số mảng (Constant Array)
Trong PHP 8, một hằng số mảng có thể được tạo bằng cách sử dụng hàm define()
<?php
define("CARS", [
"Alfa Romeo",
"BMH",
"Toyota",
"Range-Rover"
]);
var_dump(CARS);
// array(4) { [0]=> string(10) "Alfa Romeo" [1]=> string(3) "BMH" [2]=> string(6) "Toyota" [3]=> string(11) "Range-Rover" }
Hằng số tồn tại trên phạm vi toàn cục. Do đó, chúng có thể được sử dụng trong suốt tập lệnh.
Ví dụ sử dụng hằng số bên trong hàm, mặc dù chúng được định nghĩa bên ngoài hàm:
<?php
define("Hello", "what's new in PHP?");
function myTest() {
echo Hello;
}
myTest();
//output: what's new in PHP?
?>
Magic Constants trong PHP (hằng số định nghĩa trước)
Trong PHP, giá trị của một số hằng số xác định trước thay đổi tùy thuộc vào ngữ cảnh mà chúng được sử dụng. Những hằng số này được gọi là Hằng số ma thuật. Chúng không phân biệt chữ hoa chữ thường. Có chín hằng số ma thuật trong PHP, trong đó có tám hằng số ma thuật bắt đầu và kết thúc bằng dấu gạch dưới kép (__):
- __LINE__
- __FILE__
- __DIR__
- __FUNCTION__
- __CLASS__
- __TRAIT__
- __METHOD__
- __NAMESPACE__
- ClassName::class
Constant Name | Description |
__LINE__ | Trả về số dòng hiện tại |
__FILE__ | Trả về đường dẫn tuyệt đối file hiện tại |
__DIR__ | Trả về đường dẫn thư mục hiện tại |
__FUNCTION__ | Trả về function hiện tại đang gọi tới |
__CLASS__ | Trả về Class hiện tại |
__TRAIT__ | Trả về TRAIT đang sử dụng |
__METHOD__ | Trả về phương thức hiện tại |
__NAMESPACE__ | Trả về namespace hiện tại |
ClassName::class | Trả về class hiện tại |
Không giống như các hằng số thông thường, tất cả 9 hằng số này được giải quyết tại thời gian biên dịch thay vì thời gian chạy.