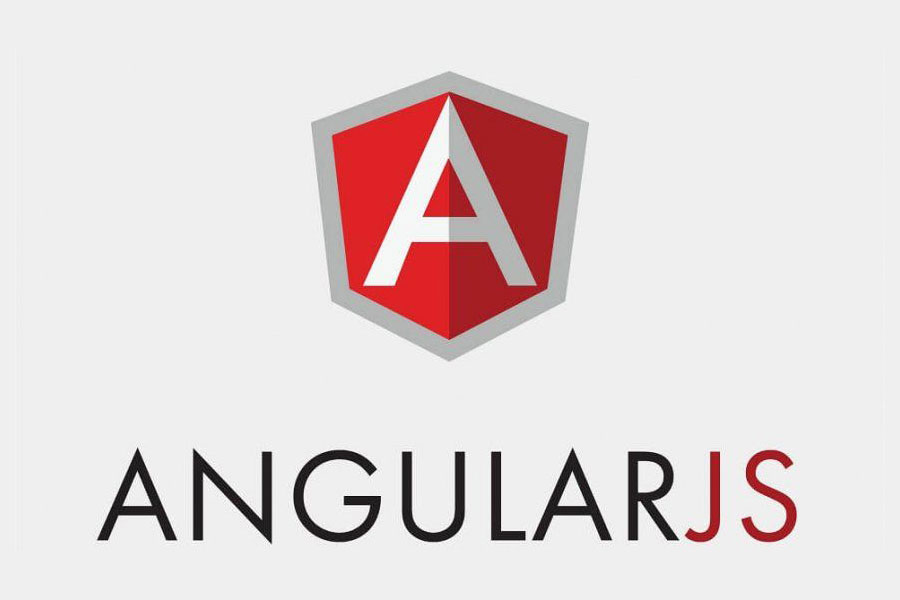
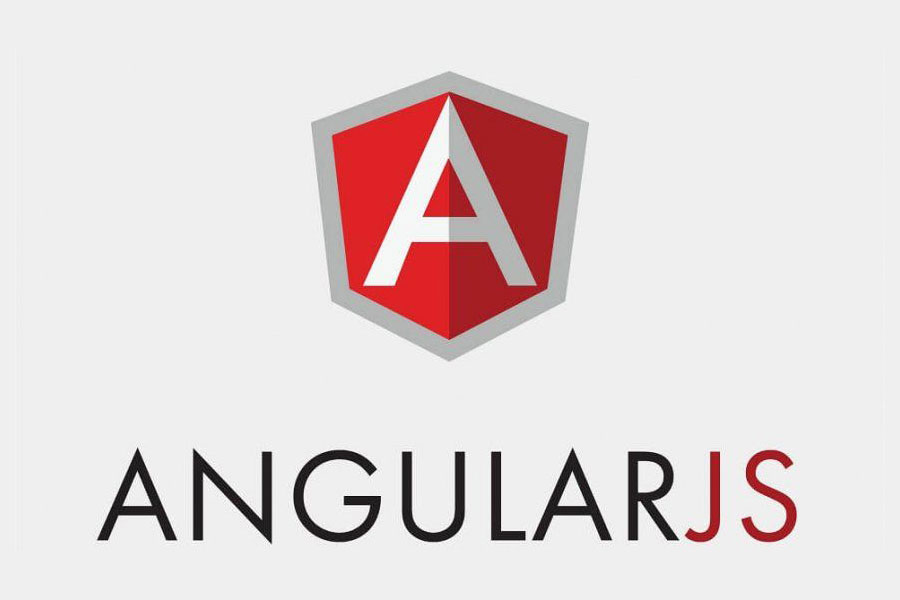
Factory và Service trong angularJS
- 29-04-2022
- Toanngo92
- 0 Comments
Ngooài việc sử dụng provider để tạo các service, AngularJS triển khai các ý tưởng thông qua kiến trúc nghiệp vụ (services). AngularJs cung cấp 2 hàm để tạo service là:
- factory()
- service()
Mục lục
Factory
Factory là một phương thức trả về giá trị, có thể sử dụng lại để thực thi các tác vụ như là validate, hay quy tắc các nghiệp vụ, factory tạo ra các giá trị mong muốn khi controller hoặc service cần nó. Những giá trị được tạo này có thể sử dụng lại cho contrrollers hoặc services bất cứ khi nào cần
Phương thức factory() là một hàm, nó tạo ra một đối tượng factory và return đối tượng đó, vì vậy nó không giống với giá trị tạo ra từ phương thức value() mình mô tả phía trên, factory sử dụng function giúp làm các nghiệp vụ phức tạp hơn như tính toán giá trị … xây dựng logic return giá trị, sau đó return giá trị về và có thể tiêm tương tự như phương thức value()
Ví dụ về factory
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Example Factory</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.js"></script>
<style>
input,
select {
margin-bottom: 10px;
}
</style>
</head>
<body ng-app="myApp">
<div ng-controller="myCtrl">
<h1>Calculate total product</h1>
Product price:
<br />
<input type="number" ng-model="price" />
<br />
Shipping type:
<br />
<select ng-model="shippingtype">
<option value="flat">Flat shipping - 10$</option>
<option value="custom">Express - custom price</option>
</select>
<br />
<input type="number" ng-model="shipping" ng-show="shippingtype == 'custom'" />
<br />
<p>Total: {{calculate()}}</p>
</div>
</body>
<script>
var myApp = angular.module('myApp', []);
myApp.factory("calculateOrderService", function () {
//define factory varialble
var factory = {};
factory.flatShipping = function (price) {
// shipping price value is 10
return price + 10;
}
factory.customShipping = function (price, shipping) {
// shipping price value is 20
return price + shipping;
}
return factory;
});
myApp.controller("myCtrl", function ($scope, calculateOrderService) {
$scope.price = 0;
$scope.shipping = 0;
$scope.total = 0;
$scope.shippingtype = "flat";
$scope.calculate = function () {
if ($scope.shippingtype == "flat") {
$scope.total = calculateOrderService.flatShipping($scope.price);
} else {
$scope.total = calculateOrderService.customShipping($scope.price, $scope.shipping);
}
return $scope.total;
};
});
</script>
</html>
Service
Trong angularJS, service tham chiếu tới một đối tượng singleton, lưu trữ một tập hợp các hàm có thể tiêm vào controoller, những hàm này có logic để giúp service có thể xử lý công việc,ví dụ service $http sử dụng để tiêm vào controller để làm các nghiệp vụ về HTTP
Để tạo ra một service trong module,AngularJS cung cấp phương thức service(), với phương thức này, chúng ta định nghĩa service và gán các phương thức mong muốn vào nó, Một service có hàm constructor ( khởi tạo), sử dụng từ khóa new để tạo ra đối tượng và đưa các phương thức, thuộc tính vào trong nó. Tuy nhiên, không giống factory, bên trong service sau khi định nghĩa không return giá trị, mọi thứ được đưa vào service thông qua this (ngữ cảnh chính đối tượng đó). Một service có thể được tiêm vào các service khác, filter, controller, directive …
Ví dụ khởi tạo dữ liệu thông qua service:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Example Service</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.js"></script>
</head>
<body ng-app="myApp">
<div ng-controller="myController">
<select ng-model="selectedlistOption" ng-change="alertselected()" ng-options="item as item.name for item in listOption track by item.id">
</select>
</div>
</body>
<script>
var app = angular.module('myApp', []);
app.service('myService', function () {
this.optionDefault = {id: 0, name: "Choose nothing"};
this.addListOption = function (listOption) {
listOption.unshift(this.optionDefault);
};
});
app.controller('myController', function ($scope, myService) {
// $scope.selected = "";
$scope.listOption = [
{id: 1, name: "Option 1"},
{id: 2, name: "Option 2"},
{id: 3, name: "Option 3"},
{id: 4, name: "Option 4"},
];
myService.addListOption($scope.listOption);
$scope.selectedlistOption= $scope.listOption[0];
$scope.alertselected = function(){
console.log($scope.selectedlistOption);
}
});
</script>
</html>
Với ví dụ trên, mình làm công việc sử dụng service thông qua cơ chế tiêm, đưa một option mặc định select vào trong danh sách select vừa khởi tạo và chọn nó làm selected mặc định.
Sự khác biệt giữa Service và Factory
Sự khác biệt | Factory | Service |
Kiểu funtion (function type) | Là một hàm, trả về đối tượng hoặc giá trị | Là một hàm khởi tạo (constructor function), hoặc khởi tạo thông qua từ khóa new |
Sử dụng (Use) | Sử dụng cho service không cấu hình, thường hay sử dụng trong cấu trúc logic phức tạp | Phù hợp sử dụng trong cấu trúc logic đơn giản |
Đặc tính (Property) | định nghĩa không sử dụng từ khóa this | Sử dụng từ khóa this |
Cơ chế tiêm thân thiện (Friendly Injection) | Không hỗ trợ | Hỗ trợ |
Primitives (nguyên thủy) | Có | Không |
Lựa chọn ưu tiên (Preferable choice) | Ưu tiên khi định nghĩa class | Ưu tiên khi định nghĩa ra các tiện ích nhanh chóng, hoặc định nghĩa hàm chuẩn ES6 |