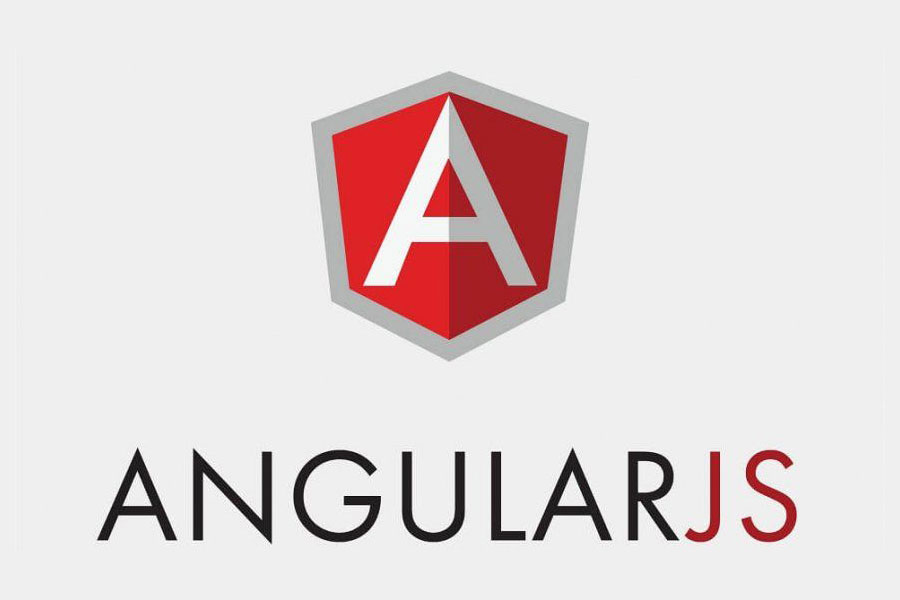
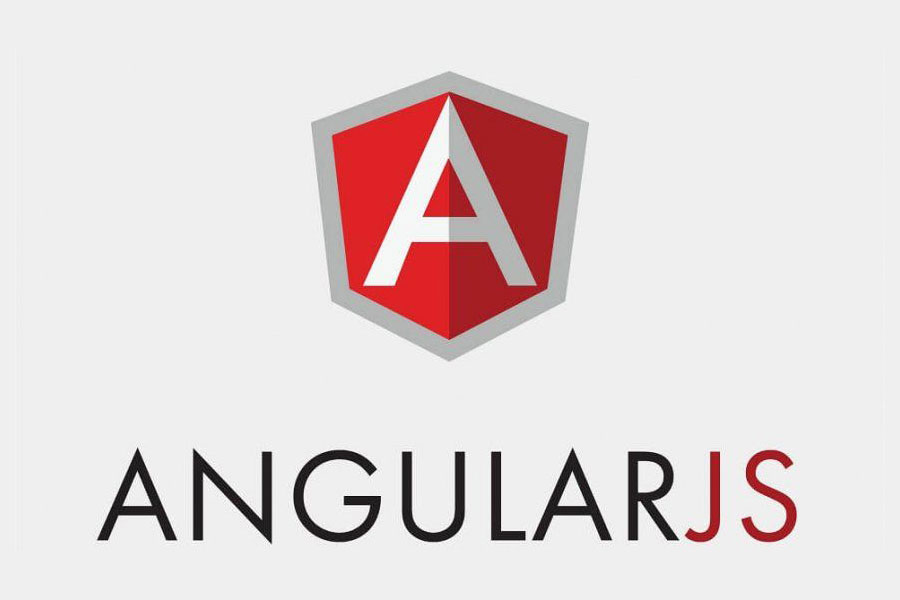
Event handling (xử lý xự kiện) trong angularJS
- 20-04-2022
- Toanngo92
- 0 Comments
Với javascript thuần hay jquery, chúng ta thường tương tác với DOM, như bắt các sự kiện click, di chuột, submit form, sự kiện thay đổi của thẻ, angularJS cung cấp cho chúng ta giải pháp ngắn gọn hơn giúp chúng ta xử lý các vấn đề này. Một số directives dùng cho event handling trong angularJS:
- ng-click
- ng-dbl-click
- ng-keydown
- ng-keyup
- ng-mousedown
- ng-mouseenter
- ng-mouseleave
- ng-change
- ng-submit
- …
Xem thêm docs tại đây: https://docs.angularjs.org/api/ng/directive/
Chúng ta chỉ cần đơn giản đưa directive event handling vào thẻ chúng ta mong muốn bắt sự kiện và định nghĩa hàm để sử dụng. Xem ví dụ dưới:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello world angular</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
</head>
<body>
<div ng-app="calculateApp" ng-controller="calculateController">
<label>Quantity:</label>
<input type="number" min="1" max="10" ng-model="qty" />
<label>Price</label>
<input type="number" min="0" max="5000" ng-model="price" />
<div>Total: {{total | currency: '$ '}}</div>
<button ng-click="calculate()">Calculate</button>
</div>
<script>
var app = angular.module('calculateApp', []);
app.controller('calculateController',function($scope){
$scope.qty = 0;
$scope.price = 0;
$scope.total = 0;
$scope.calculate = function(){
this.total = this.qty * this.price;
}
});
</script>
</body>
</html>
Ở ví dụ này, chúng ta xử lý sự kiện bấm của người dùng thông qua directive ng-click, gọi tới hàm calculate(). Dưới tầng script, định nghĩa ra phương thức (method/function) calculate và xử lý logic theo ý đồ chúng ta mong muốn. Tương tự với các sự kiện còn lại.
Xét ví dụ sau:
<!DOCTYPE html>
<html lang="en" ng-app="exampleviewApp">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Example View AngularJS</title>
<script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.min.js"></script>
</head>
<body ng-controller="mainController">
<h2>Hello {{name}}</h2>
<input type="text" ng-model="name" ng-change="change()" />
{{length}}
</body>
<script>
var exampleviewApp = angular.module('exampleviewApp', []);
exampleviewApp.controller('mainController', function($scope) {
$scope.name = '';
$scope.length = 0;
$scope.change = function(){
$scope.length = $scope.name.length;
}
});
</script>
</html>
Kết quả khi chạy code:
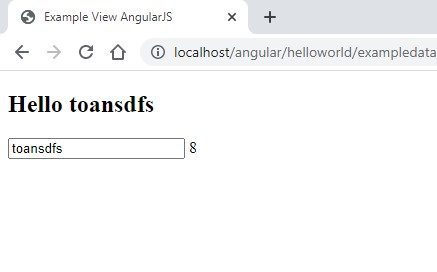
Ở ví dụ trên, chúng ta sẽ thấy giải pháp khi kết hợp data binding với sự kiện change, code rất sạch, ngắn và data được cập nhật realtime.
Bài tập
Khởi tạo một ứng dụng với giao diện như hình dưới với yêu cầu:
- Tạo ra 3 model tại 3 controller khác nhau mỗi model biểu diễn đầy đủ thông tin user
- Khi người dùng bấm và nút ở menu, đổi giao diện ở menu hiển thị thành người dùng khác (phần này có thể sử dụng js thuần)
- Khi người dùng bấm vào nút follow, trạng thái của nút đổi thành màu đỏ, text đổi thành Followed