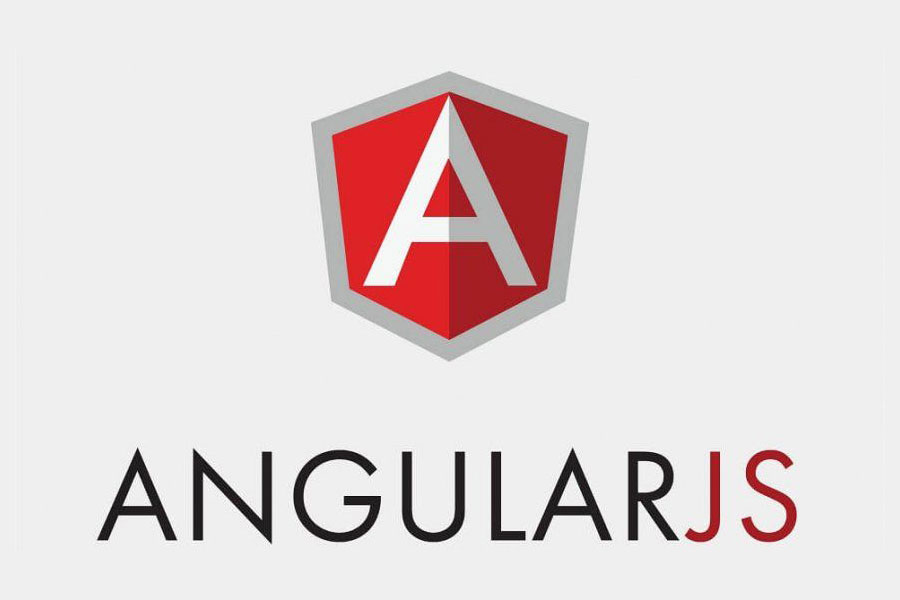
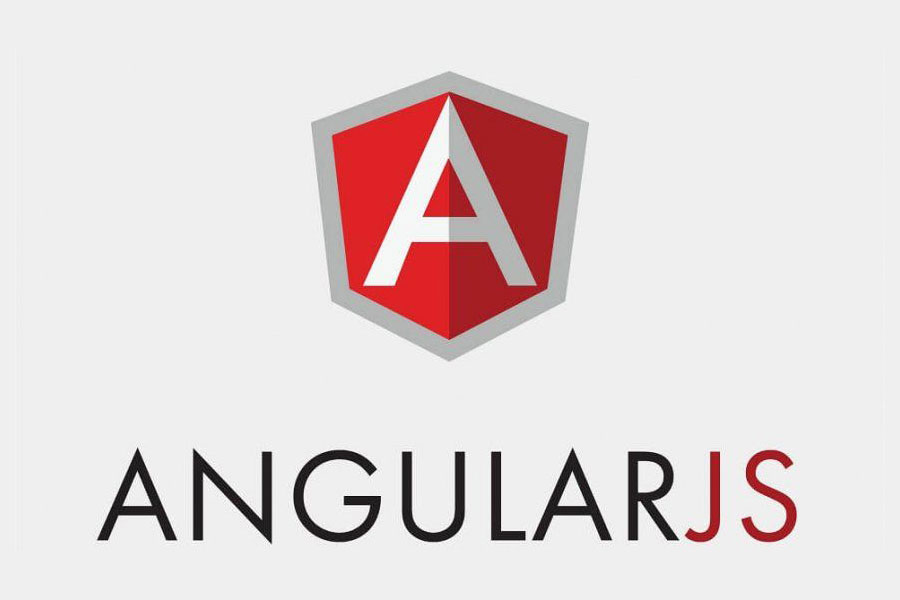
Factory and Service in angularJS
- 25-07-2022
- Toanngo92
- 0 Comments
In addition to using providers to create services, AngularJS implements ideas through a business architecture (services). AngularJs provides 2 functions to create services:
- factory()
- service()
Mục lục
Factory
A factory is a reusable, value-returning method that performs tasks such as validation, or business rules, the factory generates the desired values when the controller or service needs it. These generated values can be reused for contrrollers or services whenever needed
The factory() method is a function, it creates a factory object and returns that object, so it’s not the same as the value generated from the value() method I described above, the factory uses a function to help do this. more complex operations like value calculation… build logic that returns value, then returns value and can inject similar to value() method
Factory example
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example Factory</title> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.js"></script> <style> input, select { margin-bottom: 10px; } </style> </head> <body ng-app="myApp"> <div ng-controller="myCtrl"> <h1>Calculate total product</h1> Product price: <br /> <input type="number" ng-model="price" /> <br /> Shipping type: <br /> <select ng-model="shippingtype"> <option value="flat">Flat shipping - 10$</option> <option value="custom">Express - custom price</option> </select> <br /> <input type="number" ng-model="shipping" ng-show="shippingtype == 'custom'" /> <br /> <p>Total: {{calculate()}}</p> </div> </body> <script> var myApp = angular.module('myApp', []); myApp.factory("calculateOrderService", function () { //define factory varialble var factory = {}; factory.flatShipping = function (price) { // shipping price value is 10 return price + 10; } factory.customShipping = function (price, shipping) { // shipping price value is 20 return price + shipping; } return factory; }); myApp.controller("myCtrl", function ($scope, calculateOrderService) { $scope.price = 0; $scope.shipping = 0; $scope.total = 0; $scope.shippingtype = "flat"; $scope.calculate = function () { if ($scope.shippingtype == "flat") { $scope.total = calculateOrderService.flatShipping($scope.price); } else { $scope.total = calculateOrderService.customShipping($scope.price, $scope.shipping); } return $scope.total; }; }); </script> </html>
Service
In angularJS, service refers to a singleton object, which stores a set of functions that can be injected into the controller, these functions have logic to help the service handle the job, for example the $http service is used to inject controller to do HTTP operations
To create a service in the module, AngularJS provides the service() method, with this method we define the service and assign the desired methods to it, A service has a constructor function (initialization), using the word new key to create object and put methods and properties in it. However, unlike factory, inside service after definition does not return value, everything is injected into the service through this (the object context itself). A service can be injected into other services, filters, controllers, directives…
Example of initializing data through service:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Example Service</title> <script src="http://ajax.googleapis.com/ajax/libs/angularjs/1.2.15/angular.js"></script> </head> <body ng-app="myApp"> <div ng-controller="myController"> <select ng-model="selectedlistOption" ng-change="alertselected()" ng-options="item as item.name for item in listOption track by item.id"> </select> </div> </body> <script> var app = angular.module('myApp', []); app.service('myService', function () { this.optionDefault = {id: 0, name: "Choose nothing"}; this.addListOption = function (listOption) { listOption.unshift(this.optionDefault); }; }); app.controller('myController', function ($scope, myService) { // $scope.selected = ""; $scope.listOption = [ {id: 1, name: "Option 1"}, {id: 2, name: "Option 2"}, {id: 3, name: "Option 3"}, {id: 4, name: "Option 4"}, ]; myService.addListOption($scope.listOption); $scope.selectedlistOption= $scope.listOption[0]; $scope.alertselected = function(){ console.log($scope.selectedlistOption); } }); </script> </html>
With the above example, I do the work using the service through the injection mechanism, put a default select option in the newly initialized select list and select it as the default selected.
Difference between Service and Factory
Difference | Factory | Service |
Function type (function type) | As a function, returns an object or a value | Is a constructor function, or initialized via the new . keyword |
Use (Use) | Used for unconfigured service, often used in complex logical structure | Suitable for use in simple logical structures |
Properties | definition without using this keyword | Use this keyword |
Friendly Injection mechanism | No support | Support |
Primitives (primitives) | Have | No |
Preferred choice | Priority when defining class | Prefer when defining quick utilities, or defining ES6 standard functions |